JavaScript: Find longest substring in a given string without repeating characters
JavaScript Function: Exercise-26 with Solution
Write a JavaScript function to find the longest substring in a given string without repeating characters.
Sample Solution-1:
JavaScript Code:
// Function to find the longest substring without repeating characters
function longest_substring_without_repeating_characters(input) {
// Split the input string into an array of characters
var chars = input.split('');
var curr_char;
var str = "";
var longest_string = "";
var hash = {};
// Loop through each character in the input string
for (var i = 0; i < chars.length; i++) {
curr_char = chars[i];
// Check if the character is not already in the hash table
if (!hash[chars[i]]) {
// Add the character to the current substring
str += curr_char;
// Update the hash table with the character and its index
hash[chars[i]] = {index:i};
} else {
// If there's a repeating character, compare lengths and update if necessary
if (longest_string.length <= str.length) {
longest_string = str;
}
// Get the index of the previous occurrence of the repeating character
var prev_dupeIndex = hash[curr_char].index;
// Extract the substring from the previous occurrence to the current index
var str_FromPrevDupe = input.substring(prev_dupeIndex + 1, i);
// Update the current substring with the new non-repeating characters
str = str_FromPrevDupe + curr_char;
// Reset the hash table for the new substring
hash = {};
// Update the hash table with the characters and their indices in the new substring
for (var j = prev_dupeIndex + 1; j <= i; j++) {
hash[input.charAt(j)] = {index:j};
}
}
}
// Return the longest substring (either the current substring or the previously found longest)
return longest_string.length > str.length ? longest_string : str;
}
// Example usage:
console.log(longest_substring_without_repeating_characters("google.com"));
console.log(longest_substring_without_repeating_characters("example.com"));
Output:
gle.com xample.co
Flowchart:
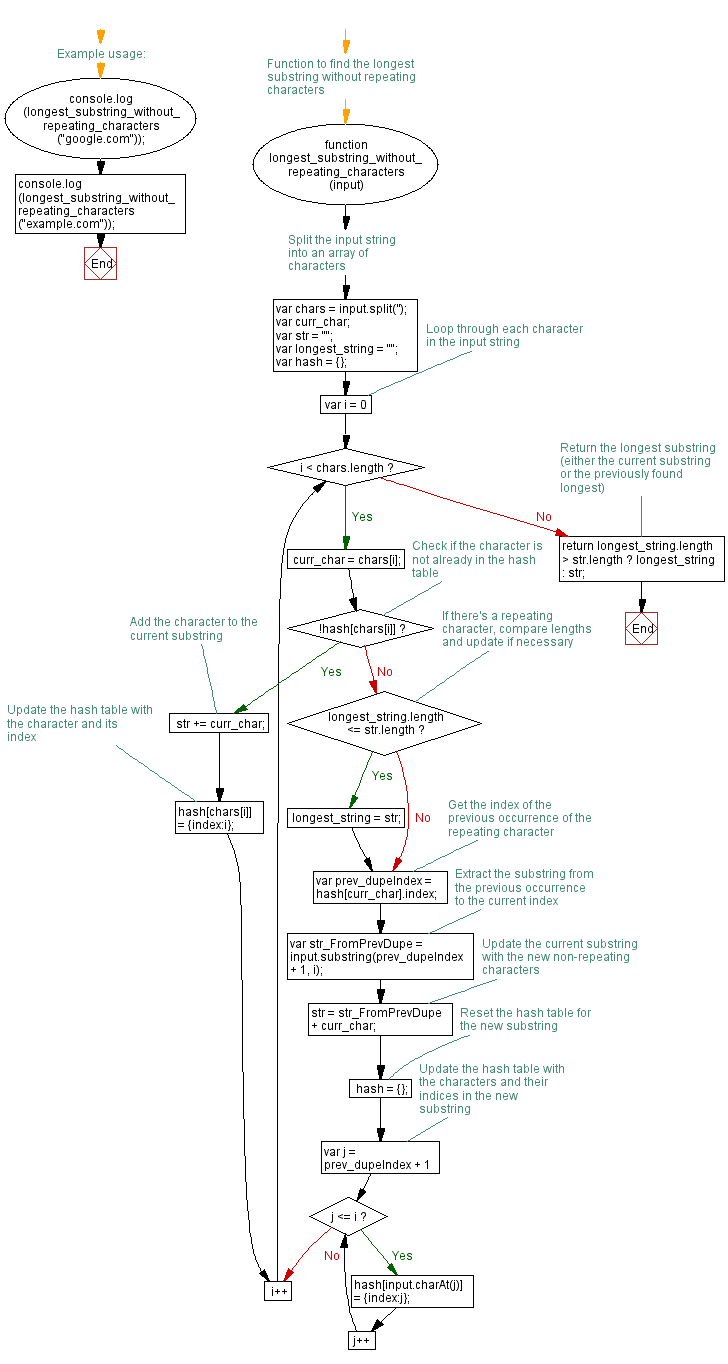
Live Demo:
See the Pen JavaScript - Find longest substring in a given a string without repeating characters-function-ex- 26 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Function to find the longest substring without repeating characters
function longest_substring_without_repeating_characters(input) {
let longestSubstring = "";
let start = 0;
let charIndexMap = {};
for (let end = 0; end < input.length; end++) {
let char = input[end];
if (charIndexMap[char] !== undefined && charIndexMap[char] >= start) {
// If repeating character found, update start index
start = charIndexMap[char] + 1;
}
// Update the index of the current character
charIndexMap[char] = end;
// Update the longest substring
let currentSubstring = input.slice(start, end + 1);
if (currentSubstring.length > longestSubstring.length) {
longestSubstring = currentSubstring;
}
}
return longestSubstring;
}
// Example usage:
console.log(longest_substring_without_repeating_characters("google.com"));
console.log(longest_substring_without_repeating_characters("example.com"));
Output:
gle.com xample.co
Flowchart:
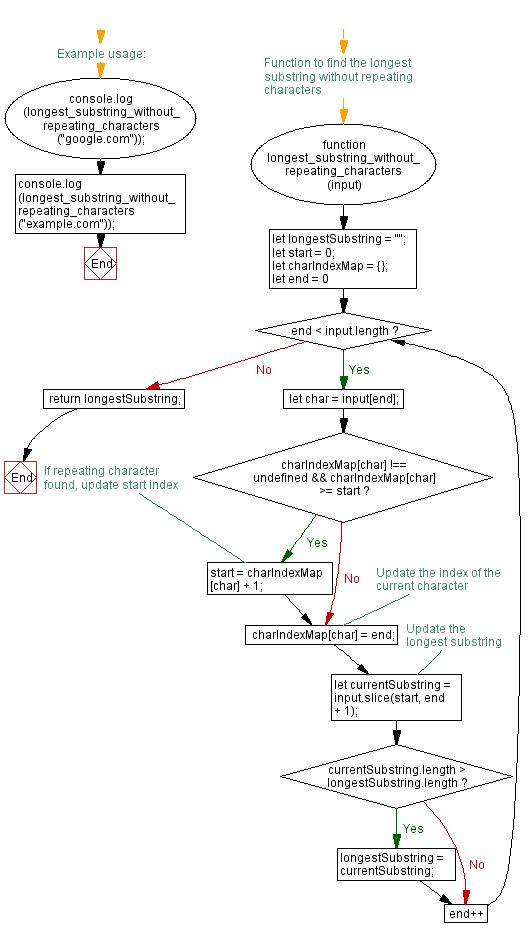
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function that accept a list of country names as input and returns the longest country name as output
Next: Write a JavaScript function that returns the longest palindrome in a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-function-exercise-26.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics