JavaScript: Accept a list of words and returns the longest
JavaScript Function: Exercise-25 with Solution
Find Longest Country Name
Write a JavaScript function that accept a list of country names as input and returns the longest country name as output.
Sample function : Longest_Country_Name(["Australia", "Germany", "United States of America"])
Expected output : "United States of America"
Visual Presentation:
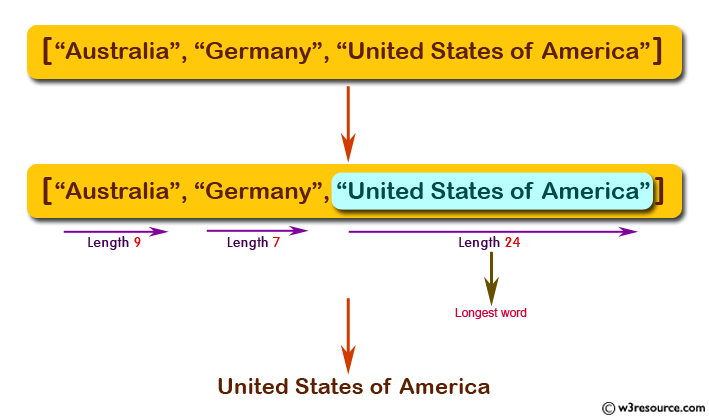
Sample Solution-1:
JavaScript Code:
// Define a function named Longest_Country_Name that finds the longest country name in an array
function Longest_Country_Name(country_name) {
// Use the reduce function to iterate through the array and find the longest country name
return country_name.reduce(function(lname, country) {
// Return the longer of the current longest name (lname) and the current country name
return lname.length > country.length ? lname : country;
}, "");
}
// Log the result of calling Longest_Country_Name with the input array to the console
console.log(Longest_Country_Name(["Australia", "Germany", "United States of America"]));
Output:
United States of America
Flowchart:
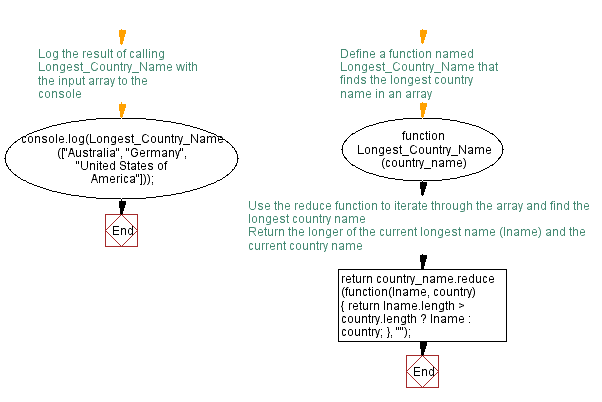
Live Demo:
See the Pen JavaScript - Bubble Sort algorithm-function-ex- 24 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Function to find the longest country name in an array
function Longest_Country_Name(country_names) {
// Check if the input array is not empty
if (country_names.length === 0) {
return "Input array is empty";
}
// Sort the array of country names based on the length of each name in descending order
const sortedNames = country_names.sort((a, b) => b.length - a.length);
// Return the first element (longest country name) after sorting
return sortedNames[0];
}
// Example usage:
// Input array of country names
var countryNames = ["Australia", "Germany", "United States of America"];
// Call the function and print the result to the console
console.log(Longest_Country_Name(countryNames));
Output:
United States of America
Flowchart:
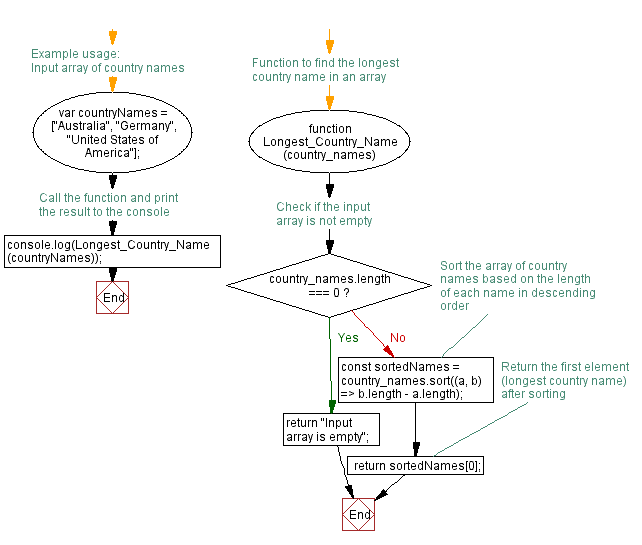
For more Practice: Solve these Related Problems:
- Write a JavaScript function that finds the longest string in an array of country names, properly handling empty arrays.
- Write a JavaScript function that compares country names by their lengths and returns the longest one, ignoring leading or trailing spaces.
- Write a JavaScript function that finds the longest country name using the reduce method to compare string lengths.
- Write a JavaScript function that returns both the longest country name and its length from an array of country names.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to apply Bubble Sort algorithm.
Next: Write a JavaScript function to find longest substring in a given a string without repeating characters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics