JavaScript: Bubble Sort algorithm
JavaScript Function: Exercise-24 with Solution
Write a JavaScript function to apply the Bubble Sort algorithm.
Note : According to wikipedia "Bubble sort, sometimes referred to as sinking sort, is a simple sorting algorithm that works by repeatedly stepping through the list to be sorted, comparing each pair of adjacent items and swapping them if they are in the wrong order".
Sample array: [12, 345, 4, 546, 122, 84, 98, 64, 9, 1, 3223, 455, 23, 234, 213]
Expected output: [3223, 546, 455, 345, 234, 213, 122, 98, 84, 64, 23, 12, 9, 4, 1]
Step by step pictorial presentation:
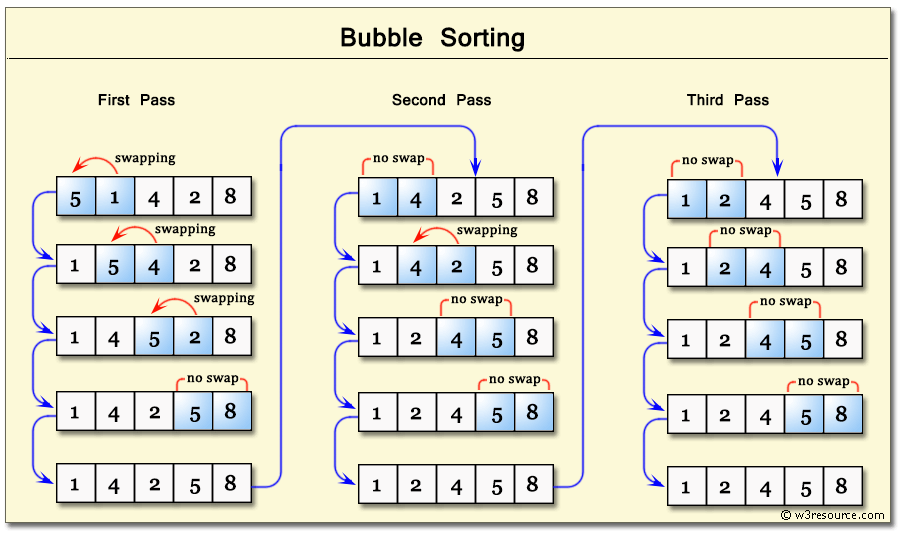
Sample Solution:
JavaScript Code:
// Define a function named bubble_Sort that implements the bubble sort algorithm on an array
function bubble_Sort(a) {
// Initialize variables for swapping (swapp), the array length (n), and a copy of the array (x)
var swapp;
var n = a.length - 1;
var x = a;
// Use a do-while loop to repeatedly iterate through the array until no swaps are needed
do {
// Set swapp to false at the beginning of each iteration
swapp = false;
// Iterate through the array using a for loop
for (var i = 0; i < n; i++) {
// Check if the current element is less than the next element
if (x[i] < x[i + 1]) {
// If true, perform a swap of the elements
var temp = x[i];
x[i] = x[i + 1];
x[i + 1] = temp;
// Set swapp to true to indicate that a swap has occurred
swapp = true;
}
}
// Decrement the array length for the next iteration
n--;
} while (swapp); // Continue the loop if at least one swap occurred in the previous iteration
// Return the sorted array
return x;
}
// Log the result of calling bubble_Sort with the input array to the console
console.log(bubble_Sort([12, 345, 4, 546, 122, 84, 98, 64, 9, 1, 3223, 455, 23, 234, 213]));
Output:
[3223,546,455,345,234,213,122,98,84,64,23,12,9,4,1]
Flowchart:
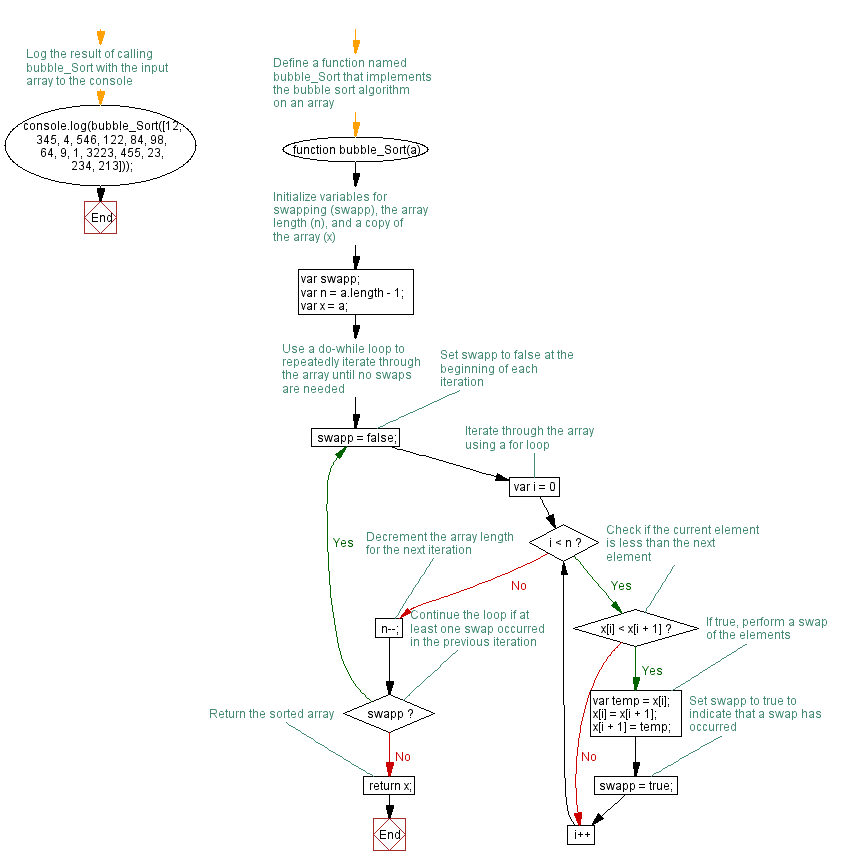
Live Demo:
See the Pen javascript-function-exercise-24 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to find the first not repeated character.
Next: Write a JavaScript function that accept a list of country names as input and returns the longest country name as output
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-function-exercise-24.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics