JavaScript: Letter count within a string
JavaScript Function: Exercise-22 with Solution
Count Letter in String
Write a JavaScript function that accepts two arguments, a string and a letter and the function will count the number of occurrences of the specified letter within the string.
Sample arguments: 'w3resource.com', 'o'
Expected output: 2
Pictorial Presentation:
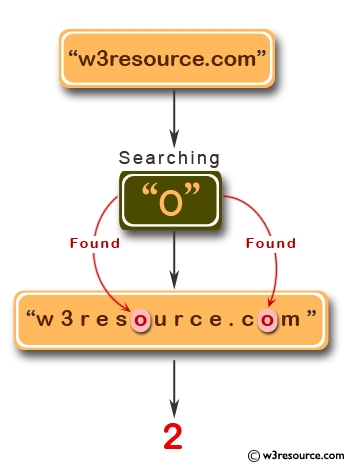
Sample Solution-1:
JavaScript Code:
// Define a function named char_count that counts the occurrences of a specified letter in a given string
function char_count(str, letter) {
// Initialize a variable letter_Count to store the count of occurrences
var letter_Count = 0;
// Iterate through each position in the input string
for (var position = 0; position < str.length; position++) {
// Check if the character at the current position is equal to the specified letter
if (str.charAt(position) == letter) {
// If true, increment the letter_Count by 1
letter_Count += 1;
}
}
// Return the final count of occurrences
return letter_Count;
}
// Log the result of calling char_count with the input string 'w3resource.com' and the letter 'o' to the console
console.log(char_count('w3resource.com', 'o'));
Output:
2
Flowchart:
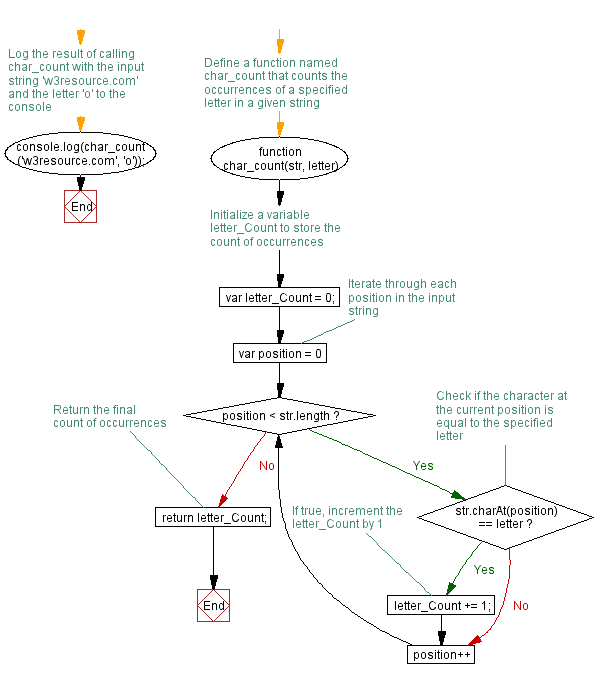
Live Demo:
See the Pen JavaScript - Letter count within a string-function-ex- 22 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Alternate function to count occurrences of a letter in a string
function countOccurrences(str, letter) {
// Use split to convert the string into an array of characters
const charArray = str.split('');
// Use filter to create an array containing only the target letter
const occurrencesArray = charArray.filter(char => char === letter);
// Return the length of the occurrences array, which is the count of occurrences
return occurrencesArray.length;
}
// Example usage:
const inputString = 'w3resource.com';
const targetLetter = 'o';
const resultCount = countOccurrences(inputString, targetLetter);
// Log the result to the console
console.log(resultCount);
Output:
2
Flowchart:
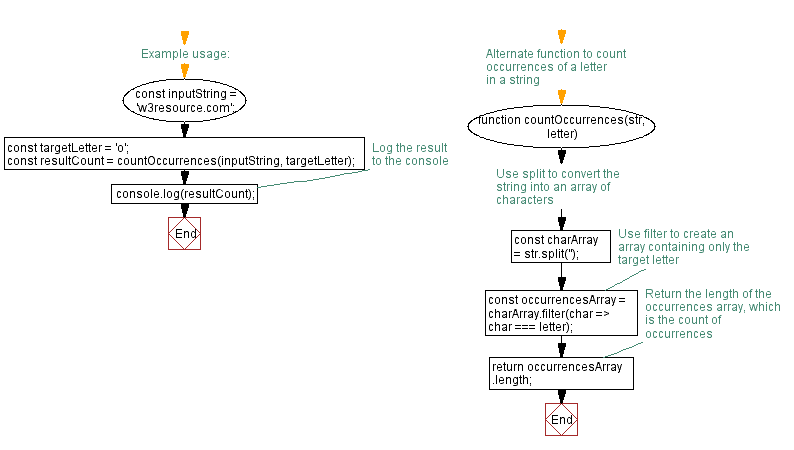
For more Practice: Solve these Related Problems:
- Write a JavaScript function that counts the occurrences of a specific letter in a string in a case-insensitive manner.
- Write a JavaScript function that uses a loop to count how many times a given letter appears in a string.
- Write a JavaScript function that employs a regular expression to count the occurrences of a letter within a string.
- Write a JavaScript function that counts the occurrences of a letter and returns a custom message if the letter is not found.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get all possible subset with a fixed length (for example 2) combinations in an array.
Next: Write a JavaScript function to find the first not repeated character.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.