JavaScript: Generates a string id (specified length) of random characters
JavaScript Function: Exercise-20 with Solution
Generate Random String ID
Write a JavaScript function that generates a string ID (specified length) of random characters.
Sample character list : "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789"
Sample Solution-1:
JavaScript Code:
// Define a function named makeid that generates a random string of specified length (l)
function makeid(l) {
// Initialize an empty string named text to store the generated random string
var text = "";
// Define a character list containing uppercase letters, lowercase letters, and digits
var char_list = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789";
// Iterate l times to generate each character in the random string
for (var i = 0; i < l; i++) {
// Append a randomly selected character from char_list to the text string
text += char_list.charAt(Math.floor(Math.random() * char_list.length));
}
// Return the generated random string
return text;
}
// Log the result of calling makeid with the parameter 8 to the console
console.log(makeid(8));
Output:
4SGqCfrz
Flowchart:
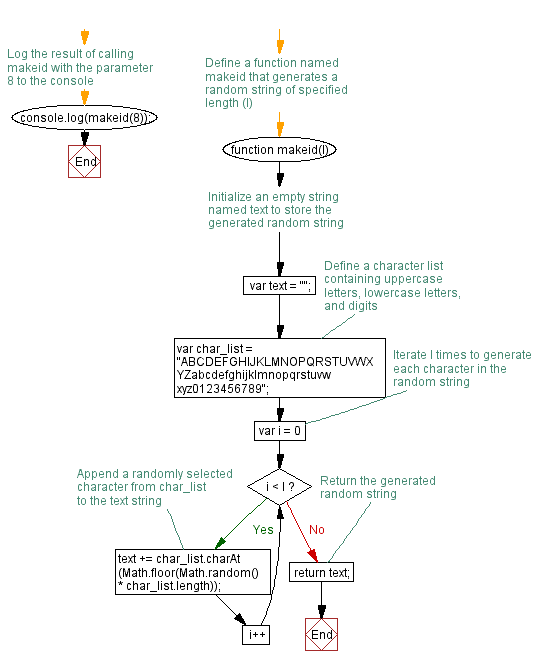
Live Demo:
See the Pen JavaScript - Generates a string id (specified length) of random characters-function-ex- 20 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Function to generate a random string ID
function generateRandomString(length) {
const characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789';
let result = '';
for (let i = 0; i < length; i++) {
// Randomly select a character from the characters string
const randomIndex = Math.floor(Math.random() * characters.length);
result += characters.charAt(randomIndex);
}
return result;
}
// Example usage:
const idLength = 10;
const randomID = generateRandomString(idLength);
// Log the generated ID to the console
console.log(randomID);
Output:
4SGqCfrz
Flowchart:
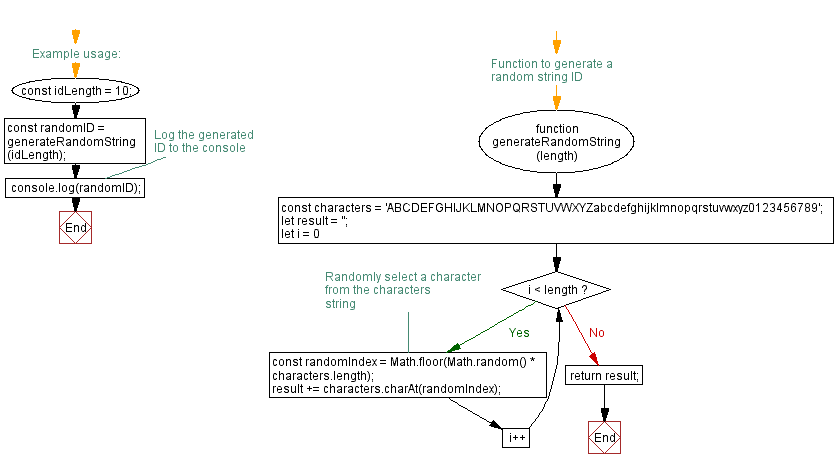
For more Practice: Solve these Related Problems:
- Write a JavaScript function that generates a random string ID of a specified length using Math.random.
- Write a JavaScript function that creates a random alphanumeric string ID by shuffling an array of characters.
- Write a JavaScript function that generates a random string ID ensuring uniqueness without using external libraries.
- Write a JavaScript function that generates a random string ID and incorporates special characters along with alphanumeric characters.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function that returns array elements larger than a number.
Next: Write a JavaScript function to get all possible subset with a fixed length (for example 2) combinations in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics