JavaScript: Bigger elements in an array
JavaScript Function: Exercise-19 with Solution
Find Elements Larger Than Number
Write a JavaScript function that returns array elements larger than a number.
Sample Solution-1:
JavaScript Code:
// Define a function named BiggerElements that returns a filtering function
function BiggerElements(val) {
// Return a filtering function that checks if the array element is greater than or equal to the given value (val)
return function(evalue, index, array) {
return (evalue >= val);
};
}
// Create an array named result by filtering elements greater than or equal to 10 using BiggerElements(10)
var result = [11, 45, 4, 31, 64, 10].filter(BiggerElements(10));
// Log the filtered array to the console
console.log(result);
Output:
[11,45,31,64,10]
Flowchart:
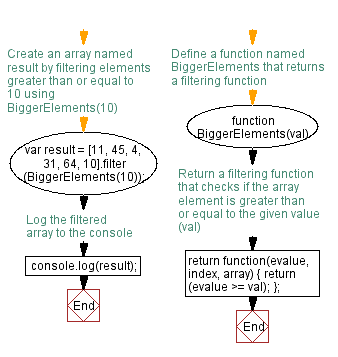
Live Demo:
See the Pen JavaScript - Bigger elements in an array-function-ex- 19 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Function to filter array elements larger than a given number
function elementsLargerThan(arr, threshold) {
// Use the Array.prototype.filter method to filter elements
const largerElements = arr.filter(element => element > threshold);
return largerElements;
}
// Example usage:
const inputArray = [1, 5, 8, 10, 15, 20];
const thresholdNumber = 8;
const resultArray = elementsLargerThan(inputArray, thresholdNumber);
// Log the result to the console
console.log(resultArray);
Output:
[10,15,20]
Flowchart:
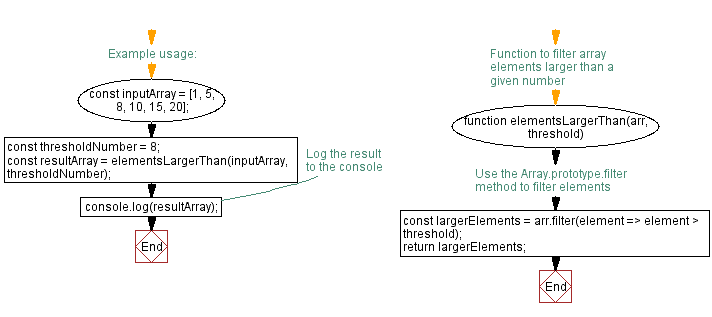
For more Practice: Solve these Related Problems:
- Write a JavaScript function that filters an array to return elements larger than a given threshold without using the filter() method.
- Write a JavaScript function that iterates through an array using a loop and returns elements greater than a specified number.
- Write a JavaScript function that finds elements larger than a given number and then sorts the resulting array in ascending order.
- Write a JavaScript function that uses recursion to find and return all array elements that are greater than a specified value.
Improve this sample solution and post your code through Disqus.
Previous: Write a function for searching JavaScript arrays with a binary search.
Next: Write a JavaScript function that generates a string id (specified length) of random characters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics