JavaScript: Number of occurrences of each letter in specified string
JavaScript Function: Exercise-17 with Solution
Count Letter Occurrences
Write a JavaScript function to get the number of occurrences of each letter in a specified string.
Visual Presentation:
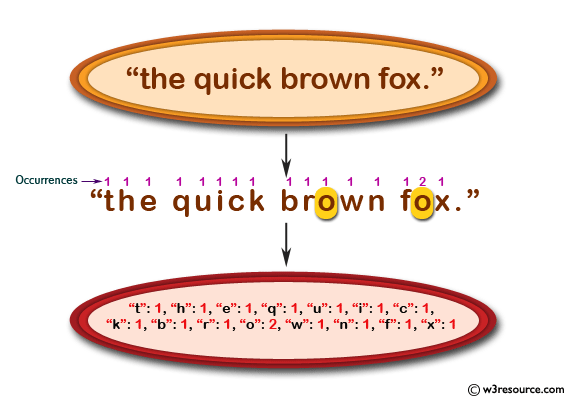
Sample Solution-1:
JavaScript Code:
// Define a function named Char_Counts that counts the occurrences of each character in the input string
function Char_Counts(str1) {
// Create an empty object uchars to store character counts
var uchars = {};
// Use the replace method to iterate through each non-whitespace character in the input string
str1.replace(/\S/g, function(l) {
// Check if the current character is already in the uchars object
uchars[l] = (isNaN(uchars[l]) ? 1 : uchars[l] + 1);
});
// Return the object containing character counts
return uchars;
}
// Log the result of calling Char_Counts with the input string "The quick brown fox jumps over the lazy dog" to the console
console.log(Char_Counts("The quick brown fox jumps over the lazy dog"));
Output:
{"T":1,"h":2,"e":3,"q":1,"u":2,"i":1,"c":1,"k":1,"b":1,"r":2,"o":4,"w":1,"n":1,"f":1,"x":1,"j":1,"m":1,"p":1,"s":1,"v":1,"t":1,"l":1,"a":1,"z":1,"y":1,"d":1,"g":1}
Flowchart:
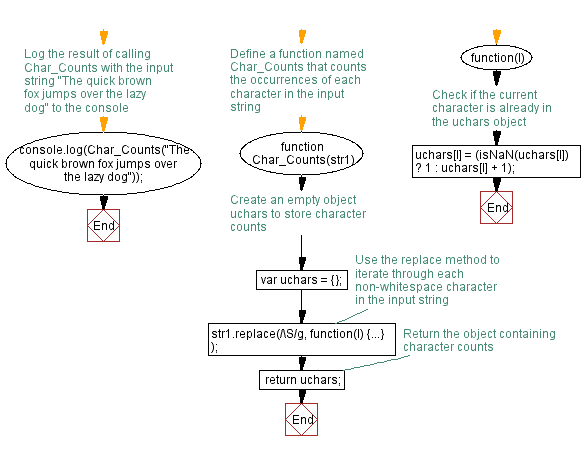
Live Demo:
See the Pen JavaScript - Number of occurrences of each letter in specified string-function-ex- 17 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Function to count occurrences of each letter in a string
function countLetterOccurrences(str) {
// Initialize an empty object to store letter occurrences
const letterOccurrences = {};
// Convert the string to lowercase to make the counting case-insensitive
const lowercaseStr = str.toLowerCase();
// Iterate through each character in the lowercase string
for (let char of lowercaseStr) {
// Check if the character is an alphabet letter
if (/^[a-z]$/.test(char)) {
// Update or initialize the count for the current letter
letterOccurrences[char] = (letterOccurrences[char] || 0) + 1;
}
}
return letterOccurrences;
}
// Example usage:
const inputString = "The quick brown fox jumps over the lazy dog.";
const result = countLetterOccurrences(inputString);
// Log the result to the console
console.log(result);
Output:
{"t":2,"h":2,"e":3,"q":1,"u":2,"i":1,"c":1,"k":1,"b":1,"r":2,"o":4,"w":1,"n":1,"f":1,"x":1,"j":1,"m":1,"p":1,"s":1,"v":1,"l":1,"a":1,"z":1,"y":1,"d":1,"g":1}
Flowchart:
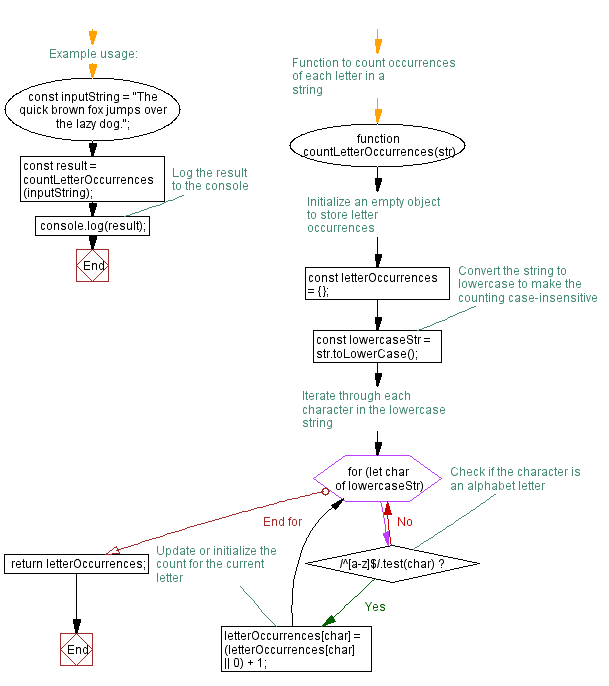
For more Practice: Solve these Related Problems:
- Write a JavaScript function that counts the occurrences of each letter in a string and returns an object mapping letters to counts.
- Write a JavaScript function that counts letter occurrences in a string while ignoring case and special characters.
- Write a JavaScript function that uses the array reduce method to count how many times each letter appears in a string.
- Write a JavaScript function that counts letter occurrences and identifies the most frequently occurring letter along with its count.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to extract unique characters from a string.
Next: Write a function for searching JavaScript arrays with a binary search.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics