JavaScript: Compute the value of bn where n is the exponent and b is the bases
JavaScript Function: Exercise-15 with Solution
Compute Power (bⁿ)
Write a JavaScript function to compute the value of bn where n is the exponent and b is the base. Accept b and n from the user and display the results.
Sample Solution-1:
JavaScript Code:
// Define a function named exp that calculates the result of raising a base (b) to a power (n)
function exp(b, n) {
// Initialize a variable ans to 1, which will store the result of the exponentiation
var ans = 1;
// Iterate from 1 to n (inclusive) to perform the exponentiation
for (var i = 1; i <= n; i++) {
// Multiply the current value of ans by the base (b)
ans = b * ans;
}
// Return the final result of the exponentiation
return ans;
}
// Log the result of calling exp with the base 2 and exponent 3 to the console
console.log(exp(2, 3));
Output:
8
Flowchart:
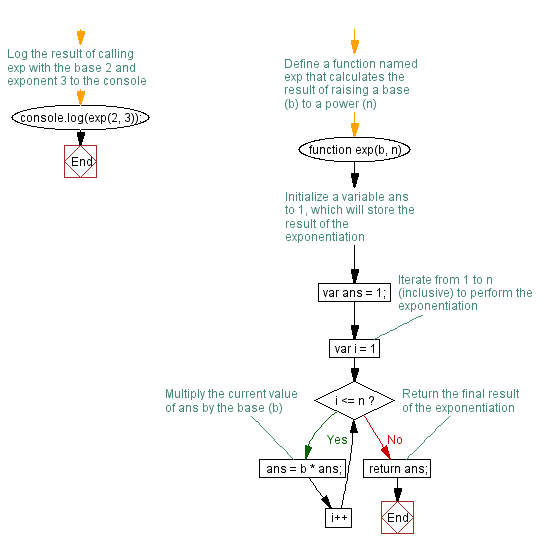
Live Demo:
See the Pen JavaScript - Compute the value of bn where n is the exponent and b is the bases-function-ex- 15 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Define a function to calculate the exponentiation of a base raised to a power
function calculateExponent(base, exponent) {
// Using the Math.pow() method to calculate the exponentiation
const result = Math.pow(base, exponent);
return result; // Return the result of the exponentiation
}
// Example usage with user input
// Prompt the user to enter the base value (b) and convert it to a floating-point number
const userBase = parseFloat(prompt("Enter the base (b):"));
// Prompt the user to enter the exponent value (n) and convert it to an integer
const userExponent = parseInt(prompt("Enter the exponent (n):"));
// Check if the user inputs are valid numeric values
if (!isNaN(userBase) && !isNaN(userExponent)) {
// Call the calculateExponent function with user input and store the result
const result = calculateExponent(userBase, userExponent);
// Display the result of the exponentiation to the console
console.log(`The result of ${userBase}^${userExponent} is: ${result}`);
} else {
// Display an error message if the user inputs are not valid numeric values
console.log("Invalid input. Please enter valid numeric values.");
}
Output:
The result of 2^3 is: 8
Flowchart:
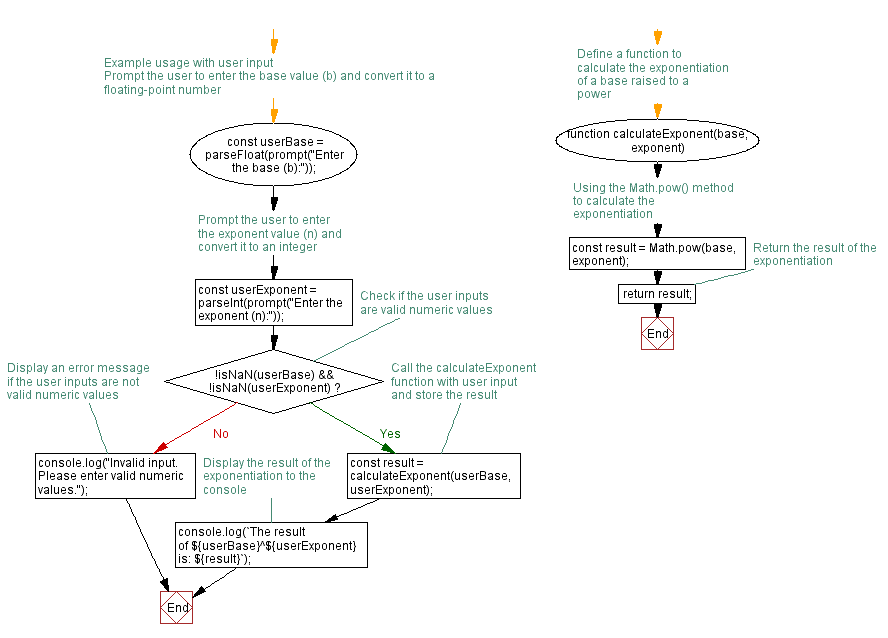
For more Practice: Solve these Related Problems:
- Write a JavaScript function that computes the power of a base raised to an exponent using a recursive method.
- Write a JavaScript function that calculates b raised to the power n iteratively without using Math.pow.
- Write a JavaScript function that implements exponentiation by squaring to compute b^n in logarithmic time.
- Write a JavaScript function that computes the power of a number and properly handles edge cases such as negative exponents.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to convert an amount to coins.
Next: Write a JavaScript function to extract unique characters from a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics