JavaScript: Find the second lowest and second greatest numbers from an array
JavaScript Function: Exercise-11 with Solution
Find Second Lowest and Greatest
Write a JavaScript function that takes an array of numbers and finds the second lowest and second greatest numbers, respectively.
Visual Presentation:
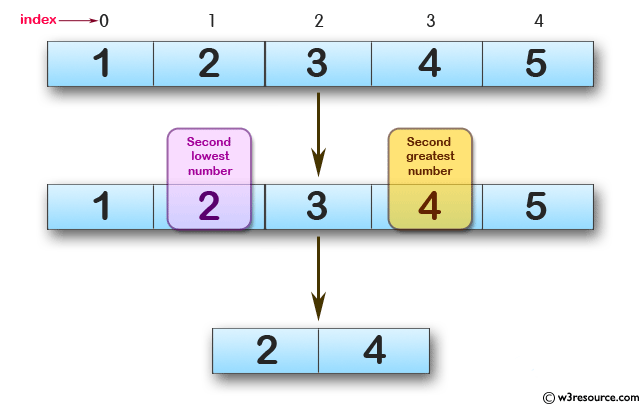
Sample Solution-1:
JavaScript Code:
// Define a function named Second_Greatest_Lowest that finds the second smallest and second largest numbers in an array
function Second_Greatest_Lowest(arr_num) {
// Sort the array in ascending order using a custom comparison function
arr_num.sort(function(x, y) {
return x - y;
});
// Initialize an array uniqa with the first element of the sorted array
var uniqa = [arr_num[0]];
// Initialize an array result to store the second smallest and second largest numbers
var result = [];
// Iterate through the sorted array to remove duplicate elements
for (var j = 1; j < arr_num.length; j++) {
if (arr_num[j - 1] !== arr_num[j]) {
uniqa.push(arr_num[j]);
}
}
// Push the second smallest and second largest numbers to the result array
result.push(uniqa[1], uniqa[uniqa.length - 2]);
// Join the result array into a string and return
return result.join(',');
}
// Log the result of calling Second_Greatest_Lowest with the input array [1,2,3,4,5] to the console
console.log(Second_Greatest_Lowest([1, 2, 3, 4, 5]));
Output:
2,4
Flowchart:
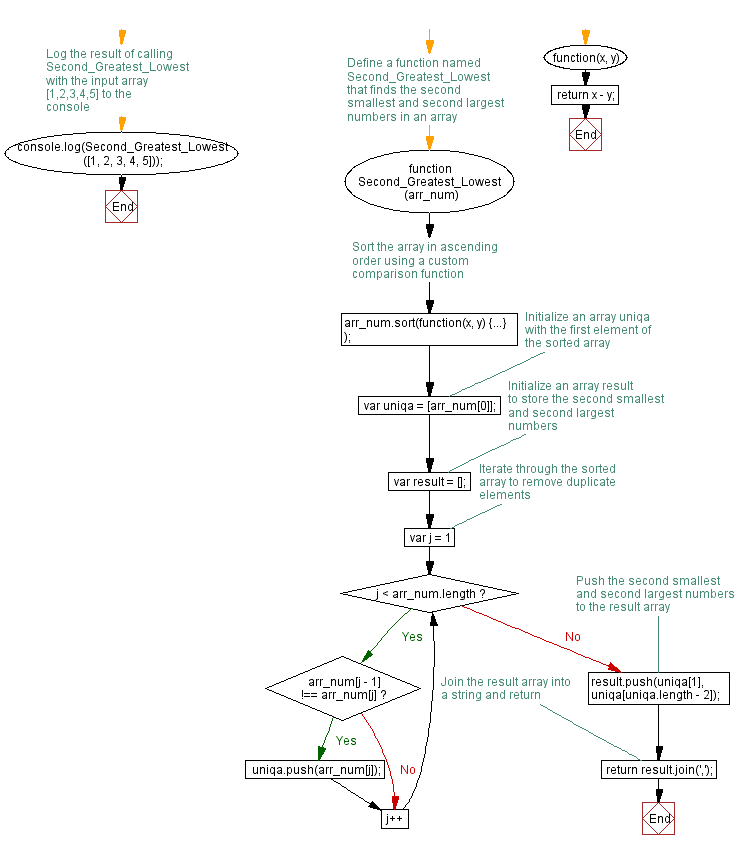
Live Demo:
See the Pen JavaScript -Second lowest and second greatest numbers from an array-function-ex- 11 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
function Second_Greatest_Lowest(arr) {
// First, sort the array in ascending order
arr.sort(function(a, b) {
return a - b;
});
// Then, get the second lowest number by accessing the index 1
let secondLowest = arr[1];
// To get the second greatest number, reverse the array and get the element at index 1
let reversedArr = arr.reverse();
let secondGreatest = reversedArr[1];
return [secondLowest, secondGreatest];
}
console.log(Second_Greatest_Lowest([1,2,3,4,5]));
Output:
2,4
Explanation:
The above function first sorts the input array in ascending order using the sort() method with a comparison function. Then, it retrieves the second lowest number in the array by accessing the element at index 1. The function reverses the sorted array to retrieve the element at index 1 of the reversed array, which is the second greatest number. Finally, the function returns an array containing the second lowest and second greatest numbers.
Flowchart:
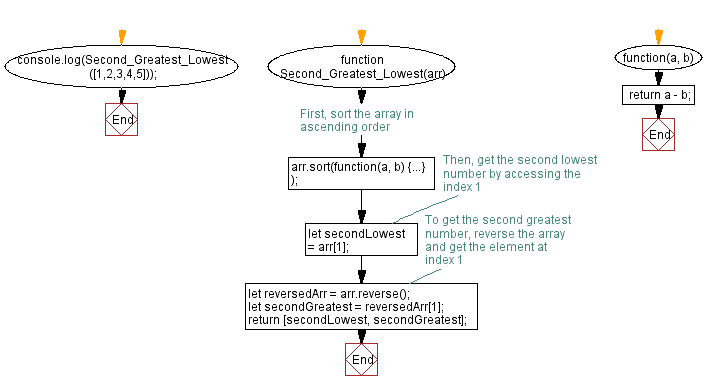
Live Demo:
See the Pen javascript-function-exercise-11-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that finds the second lowest and second highest numbers in an array without sorting it.
- Write a JavaScript function that returns the second smallest and second largest values using a single traversal of the array.
- Write a JavaScript function that identifies the second lowest and greatest numbers while properly handling duplicate values.
- Write a JavaScript function that computes the second lowest and second greatest numbers recursively.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function which returns the n rows by n columns identity matrix.
Next: Write a JavaScript function which says whether a number is perfect.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.