JavaScript: Remove items from a dropdown list
JavaScript DOM: Exercise-8 with Solution
Remove Dropdown Item
Write a JavaScript program to remove items from a drop-down list.
Sample HTML file:
<!DOCTYPE html>
<html><head>
<meta charset=utf-8 />
<title>Remove items from a dropdown list</title>
</head><body><form>
<select id="colorSelect">
<option>Red</option>
<option>Green</option>
<option>White</option>
<option>Black</option>
</select>
<input type="button" onclick="removecolor()" value="Select and Remove">
</form></body></html>
Sample Solution:
HTML Code:
<!-- Declaration of HTML document type -->
<!DOCTYPE html>
<!-- Start of HTML document -->
<html>
<!-- Start of head section -->
<head>
<!-- Internal CSS styling -->
<style type="text/css">
/* Styling body to have margin of 30px */
body {margin: 30px;}
</style>
<!-- Declaring character encoding -->
<meta charset=utf-8 />
<!-- Setting title of the document -->
<title>Remove items from a dropdown list</title>
<!-- End of head section -->
</head>
<!-- Start of body section -->
<body>
<!-- Form element containing a dropdown list -->
<form>
<select id="colorSelect">
<!-- Options in the dropdown list -->
<option>Red</option>
<option>Green</option>
<option>White</option>
<option>Black</option>
</select>
<!-- Button triggering the removecolor function on click -->
<input type="button" onclick="removecolor()" value="Select and Remove">
</form>
<!-- End of body section -->
</body>
<!-- End of HTML document -->
</html>
JavaScript Code:
// Function declaration for removecolor
function removecolor()
{
// Getting reference to the dropdown select element
var x=document.getElementById("colorSelect");
// Removing the option at the selected index
x.remove(x.selectedIndex);
}
Output:
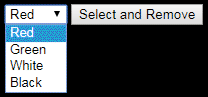
Flowchart:
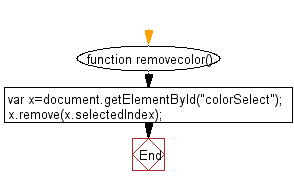
Live Demo:
See the Pen javascript-dom-exercise-8 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that removes the currently selected item from a dropdown list when a button is clicked.
- Write a JavaScript function that removes all dropdown items that meet a certain condition, such as containing a specific substring.
- Write a JavaScript function that scans a dropdown for duplicate items and removes the duplicates.
- Write a JavaScript function that removes the first dropdown option if it matches a given string input.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function that creates a table, accept row, column numbers from the user, and input row-column number as content (e.g. Row-0 Column-0) of a cell.
Next: Write a JavaScript program to count and display the items of a dropdown list, in an alert window.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.