JavaScript: Create a function to update the content of a specific cell of a table
JavaScript DOM: Exercise-6 with Solution
Update Table Cell
Write a JavaScript function that accepts a row, column (to identify a particular cell) and a string to update the cell's contents.
Sample HTML file:
<!DOCTYPE html>
<html><head>
<meta charset=utf-8 />
<title>Change the content of a cell</title>
</head><body>
<table id="myTable" border="1">
<tr><td>Row1 cell1</td>
<td>Row1 cell2</td></tr>
<tr><td>Row2 cell1</td>
<td>Row2 cell2</td></tr>
<tr><td>Row3 cell1</td>
<td>Row3 cell2</td></tr>
</table><form>
<input type="button" onclick="changeContent()" value="Change content">
</form></body></html>
Sample Solution:
HTML Code:
<!-- Declaration of HTML document type -->
<!DOCTYPE html>
<!-- Start of HTML document -->
<html>
<!-- Start of head section -->
<head>
<!-- Declaring character encoding -->
<meta charset=utf-8 />
<!-- Setting title of the document -->
<title>Change the content of a cell</title>
<!-- Internal CSS styling -->
<style type="text/css">
/* Styling body to have margin of 30px */
body {margin: 30px;}
</style>
<!-- End of head section -->
</head>
<!-- Start of body section -->
<body>
<!-- Table element with id 'myTable' and border attribute -->
<table id="myTable" border="1">
<!-- First row of the table -->
<tr><td>Row1 cell1</td>
<td>Row1 cell2</td></tr>
<!-- Second row of the table -->
<tr><td>Row2 cell1</td>
<td>Row2 cell2</td></tr>
<!-- Third row of the table -->
<tr><td>Row3 cell1</td>
<td>Row3 cell2</td></tr>
</table>
<!-- Form element containing a button triggering the changeContent function on click -->
<form>
<input type="button" onclick="changeContent()" value="Change content">
</form>
<!-- End of body section -->
</body>
<!-- End of HTML document -->
</html>
JavaScript Code:
// Function declaration for changeContent
function changeContent()
{
// Prompting the user to input the row number
rn = window.prompt("Input the Row number(0,1,2)", "0");
// Prompting the user to input the column number
cn = window.prompt("Input the Column number(0,1)","0");
// Prompting the user to input the cell content
content = window.prompt("Input the Cell content");
// Getting reference to the cells in the specified row of the table
var x=document.getElementById('myTable').rows[parseInt(rn,10)].cells;
// Setting the inner HTML content of the specified cell
x[parseInt(cn,10)].innerHTML=content;
}
Output:
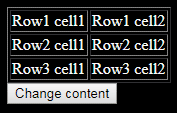
Flowchart:
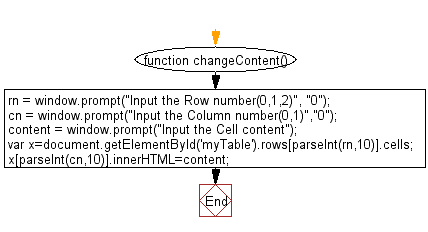
Live Demo:
See the Pen javascript-dom-exercise-6 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that updates the content of a specific cell in a table identified by its row and column indexes.
- Write a JavaScript function that changes a table cell’s content and background color when it is clicked.
- Write a JavaScript function that prompts the user for new cell content and updates the corresponding cell in the table.
- Write a JavaScript function that replaces a cell's content and logs the previous value for comparison.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to add rows to a table.
Next: Write a JavaScript function that creates a table, accept row, column numbers from the user, and input row-column number as content (e.g. Row-0 Column-0) of a cell.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.