JavaScript: Store and display the values of text boxes of a form
JavaScript DOM: Exercise-2 with Solution
Get Form Values
Write a JavaScript function to get the values of First and Last names of the following form.
Sample HTML file:
<!DOCTYPE html>
<html><head>
<meta charset=utf-8 />
<title>Return first and last name from a form - w3resource</title>
</head><body>
<form id="form1" onsubmit="getFormvalue()">
First name: <input type="text" name="fname" value="David"><br>
Last name: <input type="text" name="lname" value="Beckham"><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
Sample Solution:
HTML Code:
<!-- Declaration of HTML document type -->
<!DOCTYPE html>
<!-- Start of HTML document -->
<html>
<!-- Start of head section -->
<head>
<!-- Declaring character encoding -->
<meta charset=utf-8 />
<!-- Setting title of the document -->
<title>Return first and last name from a form - w3resource</title>
<!-- Internal CSS styling -->
<style type="text/css">
/* Styling body to have margin of 30px */
body {margin: 30px;}
</style>
<!-- End of head section -->
</head>
<!-- Start of body section -->
<body>
<!-- Creating a form with id 'form1' and onsubmit event -->
<form id="form1" onsubmit="getFormvalue()">
<!-- Input field for first name -->
First name: <input type="text" name="fname" value="David"><br>
<!-- Input field for last name -->
Last name: <input type="text" name="lname" value="Beckham"><br>
<!-- Submit button -->
<input type="submit" value="Submit">
</form>
<!-- End of body section -->
</body>
<!-- End of HTML document -->
</html>
JavaScript Code:
// Function declaration for getFormvalue
function getFormvalue()
{
// Retrieving form element with id 'form1'
var x=document.getElementById("form1");
// Looping through form elements
for (var i=0;i<x.length;i++)
{
// Checking if element value is not 'Submit'
if (x.elements[i].value!='Submit')
{
// Logging element value to console
console.log(x.elements[i].value);
}
}
}
Output:
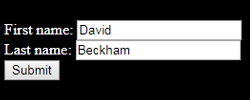
Flowchart:
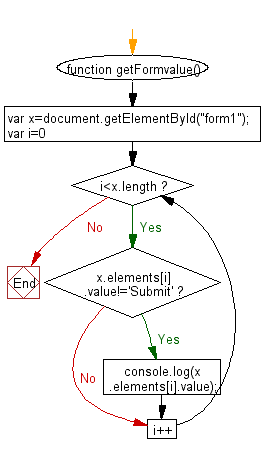
Live Demo:
See the Pen javascript-dom-exercise-2 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that retrieves the first and last name values, concatenates them, and displays the full name in an alert.
- Write a JavaScript function that prevents the default form submission and displays the form values in a designated div element.
- Write a JavaScript function that trims the input values and then logs the formatted full name to the console.
- Write a JavaScript function that updates a paragraph's text content with the first and last name values from the form upon submission.
Improve this sample solution and post your code through Disqus.
Previous: Here is a sample html file with a submit button. Now modify the style of the paragraph text through javascript code.
Next: Write a JavaScript program to set the background color of a paragraph.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.