JavaScript: Calculate the volume of a sphere
JavaScript DOM: Exercise-10 with Solution
Sphere Volume Calculator
Write a JavaScript program to calculate sphere volume.
Sample Output of the form :
Sample Solution:
HTML Code:
<!-- Declaration of HTML document type -->
<!doctype html>
<!-- Start of HTML document -->
<html lang="en">
<head>
<!-- Declaring character encoding -->
<meta charset="utf-8">
<!-- Setting title of the document -->
<title>Volume of a Sphere</title>
<!-- Internal CSS styling -->
<style>
/* Styling body to have padding at the top */
body{padding-top:30px;}
/* Styling label and input elements to display as block */
label,input{display:block;}
</style>
</head>
<!-- Start of body section -->
<body>
<!-- Paragraph explaining the purpose of the form -->
<p>Input radius value and get the volume of a sphere.</p>
<!-- Form for inputting radius and displaying volume -->
<form action="" method="post" id="MyForm">
<!-- Label and input field for entering radius -->
<label for="radius">Radius</label><input type="text" name="radius" id="radius" required>
<!-- Label and input field for displaying volume -->
<label for="volume">Volume</label><input type="text" name="volume" id="volume">
<!-- Submit button for calculating volume -->
<input type="submit" value="Calculate" id="submit">
</form>
<!-- End of body section -->
</body>
<!-- End of HTML document -->
</html>
JavaScript Code:
// Function declaration for volume_sphere
function volume_sphere()
{
// Variable to hold the volume
var volume;
// Getting the radius value from the input field
var radius = document.getElementById('radius').value;
// Taking absolute value of radius to ensure it's positive
radius = Math.abs(radius);
// Calculating the volume of the sphere
volume = (4/3) * Math.PI * Math.pow(radius, 3);
// Rounding the volume to 4 decimal places
volume = volume.toFixed(4);
// Setting the calculated volume in the volume input field
document.getElementById('volume').value = volume;
// Returning false to prevent form submission
return false;
}
// Attaching volume_sphere function to window.onload and form submit event
window.onload = document.getElementById('MyForm').onsubmit = volume_sphere;
Output:
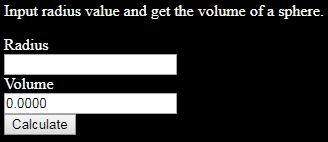
Flowchart:
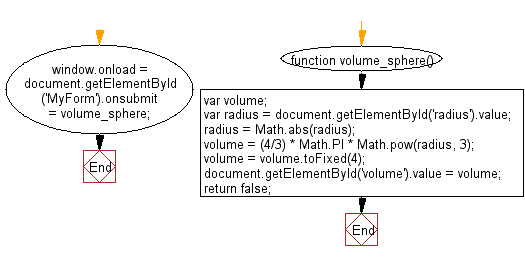
Live Demo:
See the Pen javascript-dom-exercise-10 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates the volume of a sphere using user-provided input for the radius.
- Write a JavaScript function that validates the input radius to ensure it is a positive number before calculating the sphere volume.
- Write a JavaScript function that converts the calculated sphere volume to a string formatted to two decimal places.
- Write a JavaScript function that dynamically updates a result div with the sphere's volume when a form is submitted.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to count and display the items of a dropdown list, in an alert window.
Next: Write a JavaScript program to display a random image (clicking on a button) from the following list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.