JavaScript: Check whether a date is a weekend date
JavaScript Datetime: Exercise-7 with Solution
Write a JavaScript function to test whether a date is a weekend.
Test Data :
console.log(is_weekend('Nov 15, 2014'));
console.log(is_weekend('Nov 16, 2014'));
console.log(is_weekend('Nov 17, 2014'));
Output :
"weekend"
"weekend"
undefined
Sample Solution:
JavaScript Code:
// Define a JavaScript function called is_weekend with parameter date1
var is_weekend = function(date1){
// Create a new Date object by parsing the provided date string
var dt = new Date(date1);
// Check if the day of the week is Saturday (6) or Sunday (0)
if(dt.getDay() == 6 || dt.getDay() == 0)
{
// If the day is Saturday or Sunday, return "weekend"
return "weekend";
}
}
// Output the result of checking if 'Nov 15, 2014' is a weekend
console.log(is_weekend('Nov 15, 2014'));
// Output the result of checking if 'Nov 16, 2014' is a weekend
console.log(is_weekend('Nov 16, 2014'));
// Output the result of checking if 'Nov 17, 2014' is undefined
console.log(is_weekend('Nov 17, 2014'));
Output:
weekend weekend undefined
Explanation:
In the exercise above,
- The code defines a JavaScript function named "is_weekend()" with one parameter 'date1', representing a date string.
- Inside the function:
- It creates a new Date object 'dt' by parsing the provided date string 'date1'.
- The "getDay()" method of the Date object is used to obtain the day of the week, where Sunday is represented by 0, Monday by 1, and so on.
- It checks if the day of the week obtained from "dt.getDay()" is either Saturday (6) or Sunday (0).
- If the condition is true (indicating that the day is either Saturday or Sunday), the function returns the string "weekend".
- The function does not have a return statement for cases where the provided date is not a weekend. In JavaScript, functions without an explicit return value implicitly return 'undefined'.
- The code then demonstrates the "is_weekend()" function by calling it with three different dates:
- 'Nov 15, 2014' (a Saturday)
- 'Nov 16, 2014' (a Sunday)
- 'Nov 17, 2014' (a Monday)
- Each console.log() call outputs the result of the "is_weekend()" function for the respective date to the console.
Flowchart:
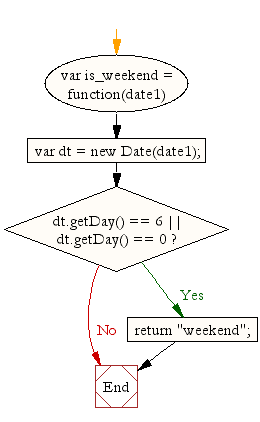
Live Demo:
See the Pen JavaScript - Check whether a date is a weekend date-date-ex- 7 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to add specified minutes to a Date object.
Next: Write a JavaScript function to get difference between two dates in days.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-date-exercise-7.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics