JavaScript: Add minutes to a Date object
JavaScript Datetime: Exercise-6 with Solution
Add Minutes to Date
Write a JavaScript function to add specified minutes to a Date object.
Test Data:
console.log(add_minutes(new Date(2014,10,2), 30).toString());
Output :
"Sun Nov 02 2014 00:30:00 GMT+0530 (India Standard Time)"
Sample Solution:
JavaScript Code:
// Define a JavaScript function called add_minutes with parameters dt and minutes
var add_minutes = function (dt, minutes) {
// Create a new Date object representing the result of adding the specified number of minutes to the provided date
return new Date(dt.getTime() + minutes*60000);
}
// Output the result of adding 30 minutes to the date "2014-11-02"
console.log(add_minutes(new Date(2014,10,2), 30).toString());
Output:
Sun Nov 02 2014 00:30:00 GMT+0530 (India Standard Time)
Explanation:
In the exercise above -
- The code defines a JavaScript function named "add_minutes(()" with two parameters: 'dt' representing a date object and 'minutes' representing the number of minutes to add to the date.
- Inside the function:
- It creates a new Date object representing the result of adding the specified number of minutes (minutes) to the provided date (dt).
- It achieves this by utilizing the "getTime()" method of the Date object, which returns the number of milliseconds since the Unix epoch (January 1, 1970 00:00:00 UTC) for the given date, and then adding the number of milliseconds corresponding to the specified minutes.
- The function returns the resulting date as a new Date object.
- The code then demonstrates the "add_minutes()" function by calling it with a specific date (November 2, 2014) and adding 30 minutes to it.
Flowchart:
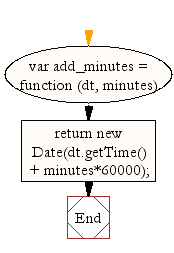
Live Demo:
See the Pen JavaScript - Add minutes to a Date object-date-ex- 6 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to compare dates (i.e. greater than, less than or equal to).
Next: Write a JavaScript function to test whether a date is a weekend.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics