JavaScript: Check if a given date is weekday, weekend
JavaScript Datetime: Exercise-55 with Solution
Check Weekday or Weekend
Write a JavaScript program to check if the current date is a weekday or a weekend.
Checks if the given date is a weekday.
- Use Date.prototype.getDay() to check weekday by using a modulo operator (%).
- Omit the argument, d, to use the current date as default.
- Use Date.prototype.getDay() to check weekend by using a modulo operator (%).
- Omit the argument, d, to use the current date as default.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function is_Weekday that checks if a given date is a weekday
const is_Weekday = (d = new Date()) => d.getDay() % 6 !== 0;
// Log a message indicating the check for the current day being a weekday
console.log("Is current day is Weekday?");
// Check if the current day is a weekday and log the result
console.log(is_Weekday());
// Define a function is_Weekend that checks if a given date is a weekend
const is_Weekend = (d = new Date()) => d.getDay() % 6 === 0;
// Log a message indicating the check for the current day being a weekend
console.log("Is current day is Weekend?");
// Check if the current day is a weekend and log the result
console.log(is_Weekend());
Output:
Is current day is Weekday? true Is current day is Weekend? false
Explanation:
In the exercise above,
- is_Weekday: This function checks whether a given date (or the current date if no date is provided) falls on a weekday (Monday to Friday). It uses the "getDay()" method to obtain the day of the week, where Sunday is represented by 0 and Saturday by 6. It returns 'true' if the day of the week is not Saturday or Sunday, indicating that it's a weekday.
- is_Weekend: This function also checks whether a given date (or the current date if no date is provided) falls on a weekend (Saturday or Sunday). Similar to "is_Weekday()", it uses the "getDay()" method to obtain the day of the week and returns 'true' if the day of the week is Saturday or Sunday.
Flowchart:
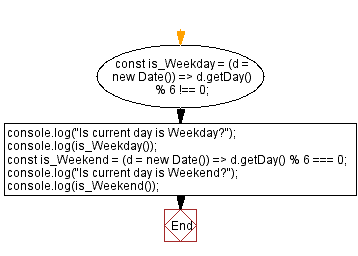
Live Demo:
See the Pen javascript-date-exercise-55 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Check if a date is between two other dates.
Next: Create and print a calendar.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics