JavaScript: Get the week start date
JavaScript Datetime: Exercise-50 with Solution
Week Start Date
Write a JavaScript function to get the week's start date.
Sample Solution:
JavaScript Code:
// Define a function startOfWeek that takes a Date object date as input
function startOfWeek(date)
{
// Calculate the difference between the date's day of the month and its day of the week
var diff = date.getDate() - date.getDay() + (date.getDay() === 0 ? -6 : 1);
// Set the date to the start of the week by setting it to the calculated difference
return new Date(date.setDate(diff));
}
// Create a new Date object representing the current date and time
dt = new Date();
// Output the start of the week for the current date and time
console.log(startOfWeek(dt).toString());
Output:
Mon Jun 18 2018 18:22:59 GMT+0530 (India Standard Time)
Explanation:
In the exercise above,
- The function "startOfWeek()" takes a "Date" object 'date' as input.
- It calculates the difference between the day of the month ('date.getDate()') and the day of the week ('date.getDay()').
- If the day of the week is Sunday (0), it adjusts the difference to ensure the start of the week is considered Monday.
- It then sets the date to the start of the week by modifying it with the calculated difference.
- Finally, it returns a new "Date" object representing the start of the week.
Flowchart:
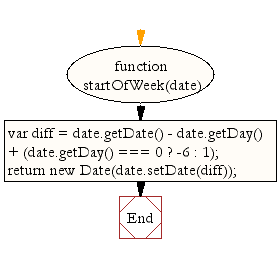
Live Demo:
See the Pen JavaScript - Get the week start date-date-ex-50 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates the start date (Monday) of the week for a given Date object.
- Write a JavaScript function that adjusts a given date to the previous Monday if it is not already Monday.
- Write a JavaScript function that returns the week start date in "MM/DD/YYYY" format.
- Write a JavaScript function that validates the date input and handles cases where the input is already the week’s start.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get time differences in years between two dates.
Next: Write a JavaScript function to get the week end date.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics