JavaScript: Comparison between two dates
JavaScript Datetime: Exercise-5 with Solution
Write a JavaScript function to compare dates (i.e. greater than, less than or equal to).
Test Data:
console.log(compare_dates(new Date('11/14/2013 00:00'), new Date('11/14/2013 00:00')));
console.log(compare_dates(new Date('11/14/2013 00:01'), new Date('11/14/2013 00:00')));
console.log(compare_dates(new Date('11/14/2013 00:00'), new Date('11/14/2013 00:01')));
Output :
"Date1 = Date2"
"Date1 > Date2"
"Date2 > Date1"
Sample Solution-1:
JavaScript Code:
var compare_dates = function(date1,date2){
if (date1>date2) return ("Date1 > Date2");
else if (date1<date2) return ("Date2 > Date1");
else return ("Date1 = Date2");
}
console.log(compare_dates(new Date('11/14/2013 00:00'), new Date('11/14/2013 00:00')));
console.log(compare_dates(new Date('11/14/2013 00:01'), new Date('11/14/2013 00:00')));
console.log(compare_dates(new Date('11/14/2013 00:00'), new Date('11/14/2013 00:01')));
Output:
Date1 = Date2 Date1 > Date2 Date2 > Date1
Flowchart:
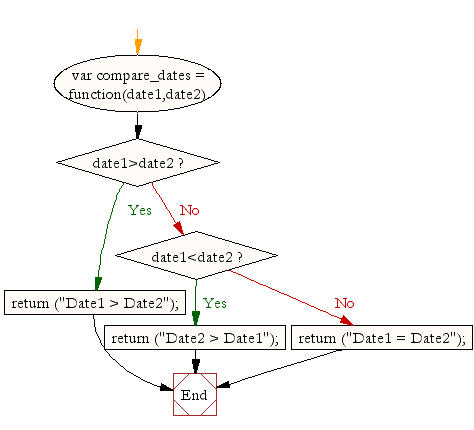
Sample Solution-2:
Checks if a date is the same as another date.
- Use Date.prototype.toISOString() and strict equality checking (===) to check if the first date is the same as the second one.
JavaScript Code:
const is_same_date = (date1, date2) => date1.toISOString() === date2.toISOString();
console.log(is_same_date(new Date(2015, 12, 15), new Date(2015, 12, 15)));
console.log(is_same_date(new Date(2015, 12, 15), new Date(2015, 12, 16)));
Output:
true false
Sample Solution-3:
Checks if a date is before another date.
- Use the less than operator (<) to check if the first date comes before the second one.
JavaScript Code:
const is_before_date = (date1, date2) => date1 < date2;
console.log("Is 2018/8/15 before 2018/8/21?")
console.log(is_before_date(new Date(2018, 8, 15), new Date(2018, 8, 21)));
console.log("Is 2018/8/21 before 2018/8/15?")
console.log(is_before_date(new Date(2018, 8, 21), new Date(2018, 8, 15)));
Output:
Is 2018/8/15 before 2018/8/21? true Is 2018/8/21 before 2018/8/15? false
Sample Solution-4:
Checks if a date is after another date.
- Use the greater than operator (>) to check if the first date comes after the second one.
JavaScript Code:
const is_after_date = (date1, date2) => date1 > date2;
console.log("Is 2018/8/15 after 2018/8/21?")
console.log(is_after_date(new Date(2018, 8, 15), new Date(2018, 8, 21)));
console.log("Is 2018/8/21 after 2018/8/15?")
console.log(is_after_date(new Date(2018, 8, 21), new Date(2018, 8, 15)));
Output:
Is 2018/8/15 after 2018/8/21? false Is 2018/8/21 after 2018/8/15? true
Live Demo:
See the Pen JavaScript - Comparison between two dates-date-ex- 5 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get the month name from a particular date.
Next: Write a JavaScript function to add specified minutes to a Date object.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-date-exercise-5.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics