JavaScript: Get time differences in years between two dates
JavaScript Datetime: Exercise-49 with Solution
Time Diff in Years
Write a JavaScript function to get time differences in years between two dates.
Test Data :
dt1 = new Date("June 13, 2014 08:11:00");
dt2 = new Date("October 19, 2017 11:13:00");
console.log(diff_years(dt1, dt2));
3
Sample Solution:
JavaScript Code:
// Define a function called diff_years that calculates the difference in years between two given dates (dt2 and dt1)
function diff_years(dt2, dt1)
{
// Calculate the difference in milliseconds between the two dates
var diff = (dt2.getTime() - dt1.getTime()) / 1000;
// Convert the difference from milliseconds to days
diff /= (60 * 60 * 24);
// Calculate the approximate number of years by dividing the difference in days by the average number of days in a year (365.25)
return Math.abs(Math.round(diff / 365.25));
}
// Create two Date objects representing different dates
dt1 = new Date(2014, 10, 2); // November 2, 2014
dt2 = new Date(2014, 10, 11); // November 11, 2014
// Output the difference in years between the two dates
console.log(diff_years(dt1, dt2));
// Create two Date objects representing different dates
dt1 = new Date("June 13, 2014 08:11:00"); // June 13, 2014
dt2 = new Date("October 19, 2017 11:13:00"); // October 19, 2017
// Output the difference in years between the two dates
console.log(diff_years(dt1, dt2));
Output:
0 3
Explanation:
In the exercise above,
- The "diff_years()" function takes two Date objects 'dt2' and 'dt1' as parameters.
- It calculates the difference in milliseconds between the two dates.
- Then, it converts the difference from milliseconds to days.
- Next, it divides the difference in days by the average number of days in a year (365.25) to approximate the number of years.
- Finally, it returns the absolute value of the rounded difference in years.
Flowchart:
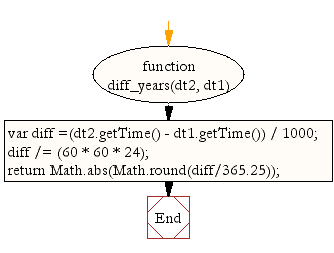
Live Demo:
See the Pen JavaScript - Get time differences in years between two dates-date-ex-49 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates the difference in years between two dates by subtracting their full year values.
- Write a JavaScript function that adjusts the year difference based on the month and day of the two dates.
- Write a JavaScript function that returns the absolute difference in years as a number.
- Write a JavaScript function that formats the year difference in a sentence, e.g., "The difference is X years."
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get time differences in months between two dates.
Next: Write a JavaScript function to get the week start date.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.