JavaScript: Get time differences in months between two dates
JavaScript Datetime: Exercise-48 with Solution
Time Diff in Months
Write a JavaScript function to get time differences in months between two dates.
Test Data:
dt1 = new Date("June 13, 2014 08:11:00");
dt2 = new Date("October 19, 2014 11:13:00");
console.log(diff_months(dt1, dt2));
5
Sample Solution:
JavaScript Code:
// Define a function called diff_months that calculates the difference in months between two given dates (dt2 and dt1).
function diff_months(dt2, dt1)
{
// Calculate the difference in milliseconds between the two dates.
var diff =(dt2.getTime() - dt1.getTime()) / 1000;
// Convert the difference from milliseconds to months by dividing it by the number of milliseconds in an hour, a day, a week, and approximately 4 weeks in a month.
diff /= (60 * 60 * 24 * 7 * 4);
// Round the result to the nearest integer using Math.round().
return Math.abs(Math.round(diff));
}
// Define two Date objects representing different dates.
dt1 = new Date(2014,10,2);
dt2 = new Date(2014,10,11);
// Calculate and log the difference in months between the two dates using the diff_months function.
console.log(diff_months(dt1, dt2));
// Define two Date objects representing different dates.
dt1 = new Date("June 13, 2014 08:11:00");
dt2 = new Date("October 19, 2014 11:13:00");
// Calculate and log the difference in months between the two dates using the diff_months function.
console.log(diff_months(dt1, dt2));
Output:
0 5
Explanation:
In the exercise above,
- The "diff_months()" function takes two Date objects, 'dt2' and 'dt1', as parameters.
- Inside the function, it calculates the difference in milliseconds between the two dates using the "getTime()" method.
- It then converts the difference from milliseconds to months by dividing it successively by the number of milliseconds in an hour, a day, a week, and approximately 4 weeks in a month.
- The result is rounded to the nearest integer using "Math.round()" to get the whole number of months.
- Finally, the absolute value of the rounded difference is returned.
Flowchart:
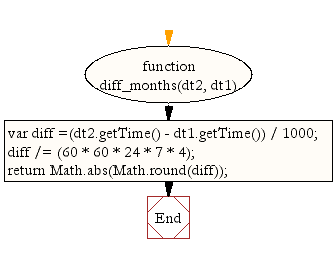
Live Demo:
See the Pen JavaScript - Get time differences in months between two dates-date-ex-48 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates the difference in months between two dates by comparing year and month values.
- Write a JavaScript function that adjusts for the day differences when computing the month difference.
- Write a JavaScript function that returns the month difference as an integer, rounding down partial months.
- Write a JavaScript function that validates the input dates and computes the total months difference combining year and month parts.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get time differences in weeks between two dates.
Next: Write a JavaScript function to get time differences in years between two dates.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics