JavaScript: Get time differences in hours between two dates
JavaScript Datetime: Exercise-45 with Solution
Write a JavaScript function to get time differences in hours between two dates.
Test Data:
dt1 = new Date("October 13, 2014 08:11:00");
dt2 = new Date("October 13, 2014 11:13:00");
console.log(diff_hours(dt1, dt2));
3
Sample Solution:
JavaScript Code:
// Define a function called diff_hours that calculates the difference in hours between two given Date objects (dt2 and dt1)
function diff_hours(dt2, dt1)
{
// Calculate the difference in milliseconds between the two provided Date objects by subtracting the milliseconds value of dt1 from the milliseconds value of dt2
var diff =(dt2.getTime() - dt1.getTime()) / 1000;
// Convert the difference from milliseconds to hours by dividing it by the number of seconds in an hour (3600)
diff /= (60 * 60);
// Return the absolute value of the rounded difference in hours
return Math.abs(Math.round(diff));
}
// Create a Date object dt1 representing November 2, 2014
dt1 = new Date(2014,10,2);
// Create a Date object dt2 representing November 3, 2014
dt2 = new Date(2014,10,3);
// Output the difference in hours between dt1 and dt2 using the diff_hours function
console.log(diff_hours(dt1, dt2));
// Create a Date object dt1 representing October 13, 2014, at 08:11:00
dt1 = new Date("October 13, 2014 08:11:00");
// Create a Date object dt2 representing October 13, 2014, at 11:13:00
dt2 = new Date("October 13, 2014 11:13:00");
// Output the difference in hours between dt1 and dt2 using the diff_hours function
console.log(diff_hours(dt1, dt2));
Output:
24 3
Explanation:
In the exercise above,
- The difference in milliseconds between 'dt2' and 'dt1' is computed by subtracting the milliseconds value of 'dt1' from the milliseconds value of 'dt2'.
- This difference is then converted to hours by dividing it by the number of seconds in an hour (3600).
- The absolute value of the rounded difference in hours is returned.
Two sets of Date objects ('dt1' and 'dt2') are created:
- The first set represents November 2, 2014, and November 3, 2014.
- The second set represents October 13, 2014, at 08:11:00 and October 13, 2014, at 11:13:00.
Flowchart:
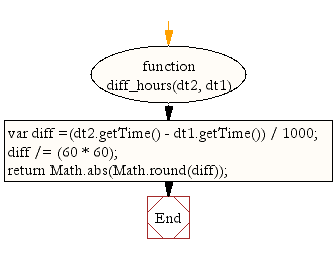
Live Demo:
See the Pen JavaScript - Get time differences in hours between two dates-date-ex-45 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get time differences in minutes between two dates.
Next: Write a JavaScript function to get time differences in days between two dates.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-date-exercise-45.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics