JavaScript: Get time differences in minutes between two dates
JavaScript Datetime: Exercise-44 with Solution
Time Diff in Minutes
Write a JavaScript function to get time differences in minutes between two dates.
Test Data :
dt1 = new Date("October 13, 2014 11:11:00");
dt2 = new Date("October 13, 2014 11:13:00");
console.log(diff_minutes(dt1, dt2));
2
Sample Solution:
JavaScript Code:
// Define a function called diff_minutes that calculates the difference in minutes between two Date objects (dt2 and dt1)
function diff_minutes(dt2, dt1)
{
// Calculate the difference in milliseconds between the two provided dates and convert it to seconds
var diff =(dt2.getTime() - dt1.getTime()) / 1000;
// Convert the difference from seconds to minutes
diff /= 60;
// Return the absolute value of the rounded difference in minutes
return Math.abs(Math.round(diff));
}
// Create a Date object dt1 representing November 2, 2014
dt1 = new Date(2014,10,2);
// Create a Date object dt2 representing November 3, 2014
dt2 = new Date(2014,10,3);
// Output the difference in minutes between dt1 and dt2 using the diff_minutes function
console.log(diff_minutes(dt1, dt2));
Output:
1440
Explanation:
In the exercise above,
- Define a function named "diff_minutes()" that calculates the difference in minutes between two given Date objects ("dt2" and "dt1").
- Inside the diff_minutes function:
- It calculates the difference in milliseconds between the two provided Date objects by subtracting the milliseconds value of 'dt1' from the milliseconds value of 'dt2'.
- This difference is then converted to seconds by dividing it by 1000.
- Next, it converts the difference from seconds to minutes by dividing it by 60.
- Finally, it rounds the resulting value to the nearest integer using "Math.round()" and returns the absolute value of this rounded difference using "Math.abs()".
- After defining the function, the code creates two Date objects:
- dt1 represents November 2, 2014.
- dt2 represents November 3, 2014.
- It then calls the "diff_minutes()" function with 'dt1' and 'dt2' as arguments to calculate the difference in minutes between these two dates.
- Finally, it outputs the calculated difference in minutes between 'dt1' and 'dt2' using console.log().
Flowchart:
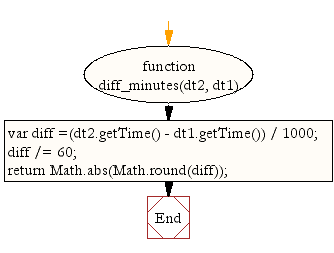
Live Demo:
See the Pen JavaScript - Get time differences in minutes between two dates-date-ex-44 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates the difference in minutes between two Date objects by subtracting their timestamps.
- Write a JavaScript function that uses Math.floor to return the whole number of minutes between two dates.
- Write a JavaScript function that accepts date strings, converts them to Date objects, and computes the minute difference.
- Write a JavaScript function that adjusts for daylight saving time when calculating minute differences.
Go to:
PREV : Add Months to Date.
NEXT : Time Diff in Hours.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.