JavaScript: Add specified months to a date
JavaScript Datetime: Exercise-43 with Solution
Add Months to Date
Write a JavaScript function to add specified months to a date.
Test Data :
dt = new Date(2014,10,2);
console.log(add_months(dt, 10).toString());
Output :
"Wed Sep 02 2015 00:00:00 GMT+0530 (India Standard Time)"
Sample Solution:
JavaScript Code:
// Define a function add_months that adds a specified number of months (n) to a given Date object (dt)
function add_months(dt, n)
{
// Set the month of the provided date to the result of adding n months to its current month
return new Date(dt.setMonth(dt.getMonth() + n));
}
// Create a new Date object representing the current date and time
dt = new Date();
// Output the result of adding 10 months to the current date and time
console.log(add_months(dt, 10).toString());
// Create a new Date object representing November 2, 2014
dt = new Date(2014,10,2);
// Output the result of adding 10 months to November 2, 2014
console.log(add_months(dt, 10).toString());
Output:
Sat Apr 20 2019 17:07:13 GMT+0530 (India Standard Time) Wed Sep 02 2015 00:00:00 GMT+0530 (India Standard Time)
Explanation:
In the exercise above,
- The code defines a function called "add_months()" that takes two parameters:
- dt: A Date object representing a specific date.
- n: The number of months to add to the provided date.
- Inside the add_months function:
- It uses the "setMonth()" method of the Date object ("dt") to modify its month component. It calculates the new month by adding 'n' months to the current month of the Date object.
- The "setMonth()" method updates the month part of the date and returns the number of milliseconds since January 1, 1970, 00:00:00 UTC, representing the updated date.
- The 'new Date()' constructor is used to create a new Date object based on the modified milliseconds value.
- After defining the function, the code creates a new Date object "dt" representing the current date and time using 'new Date()'.
- It then calls the "add_months()" function with this Date object and the number 10 as arguments to add 10 months to the current date and time.
- Finally, it outputs the result of adding 10 months to the current date and time using console.log().
- Next, the code creates another new Date object "dt" representing November 2, 2014, using 'new Date(2014,10,2)'.
- It again calls the "add_months()" function with this Date object and the number 10 as arguments to add 10 months to November 2, 2014.
- Finally, it outputs the result of adding 10 months to November 2, 2014, using console.log().
Flowchart:
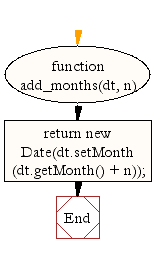
Live Demo:
See the Pen JavaScript - Add specified months to a date-date-ex-43 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that adds a specified number of months to a Date object using setMonth() and handles month overflow.
- Write a JavaScript function that adjusts the day of the month if the new month has fewer days than the original date.
- Write a JavaScript function that validates month addition and returns a formatted Date string after the update.
- Write a JavaScript function that handles edge cases like adding months to a date at the end of the month (e.g., January 31).
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to add specified weeks to a date.
Next: Write a JavaScript function to get time differences in minutes between two dates.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.