JavaScript: Add specified weeks to a date
JavaScript Datetime: Exercise-42 with Solution
Add Weeks to Date
Write a JavaScript function to add specified weeks to a date.
Test Data :
dt = new Date(2014,10,2);
console.log(add_weeks(dt, 10).toString());
Output :
"Sun Jan 11 2015 00:00:00 GMT+0530 (India Standard Time)"
Sample Solution:
JavaScript Code:
// Define a function add_weeks that adds a specified number of weeks (n) to a given Date object (dt)
function add_weeks(dt, n)
{
// Set the date of the provided date to the result of adding n weeks (7 days each) to its current date
return new Date(dt.setDate(dt.getDate() + (n * 7)));
}
// Create a new Date object representing the current date and time
dt = new Date();
// Output the result of adding 10 weeks to the current date and time
console.log(add_weeks(dt, 10).toString());
// Create a new Date object representing November 2, 2014
dt = new Date(2014,10,2);
// Output the result of adding 10 weeks to November 2, 2014
console.log(add_weeks(dt, 10).toString());
Output:
Wed Aug 29 2018 16:56:47 GMT+0530 (India Standard Time) Sun Jan 11 2015 00:00:00 GMT+0530 (India Standard Time)
Explanation:
In the exercise above,
- The code defines a function called "add_weeks()" that takes two parameters:
- dt: A Date object representing a specific date.
- n: The number of weeks to add to the provided date.
- Inside the add_weeks function:
- It uses the "setDate()" method of the Date object ("dt") to modify its date component. It calculates the new date by adding n * 7 days (representing 'n' weeks) to the current date of the Date object.
- The "setDate()" method updates the date part of the date and returns the number of milliseconds since January 1, 1970, 00:00:00 UTC, representing the updated date.
- After defining the function, the code creates a new Date object "dt" representing the current date and time using 'new Date()'.
- It then calls the "add_weeks()" function with this Date object and the number 10 as arguments to add 10 weeks to the current date and time.
- Finally, it outputs the result of adding 10 weeks to the current date and time using console.log().
- Next, the code creates another new Date object "dt" representing November 2, 2014, using new Date(2014,10,2).
- It again calls the "add_weeks()" function with this Date object and the number 10 as arguments to add 10 weeks to November 2, 2014.
- Finally, it outputs the result of adding 10 weeks to November 2, 2014, using console.log().
Flowchart:
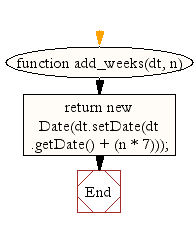
Live Demo:
See the Pen JavaScript - Add specified weeks to a date-date-ex-42 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that adds a specified number of weeks to a Date object by converting weeks to days.
- Write a JavaScript function that uses setDate() to add the equivalent days of the given number of weeks.
- Write a JavaScript function that validates the weeks parameter and returns the new date in a formatted string.
- Write a JavaScript function that handles negative week values by subtracting days from the Date object.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to add specified years to a date.
Next: Write a JavaScript function to add specified months to a date.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.