JavaScript: Get Timezone
JavaScript Datetime: Exercise-37 with Solution
Get Timezone
Write a JavaScript function to get the Timezone.
Test Data:
dt = new Date();
console.log(seconds_with_leading_zeros(dt));
"India Standard Time"
Sample Solution:
JavaScript Code:
// Define a function seconds_with_leading_zeros that takes a Date object dt as input
function seconds_with_leading_zeros(dt)
{
// Retrieve the seconds component of the current date and time and return it
// This is done by converting the current date to a string (new Date().toString()),
// then using a regular expression to extract the seconds component
return /\((.*)\)/.exec(new Date().toString())[1];
}
// Create a new Date object representing the current date and time
dt = new Date();
// Output the seconds component of the current date and time
console.log(seconds_with_leading_zeros(dt));
// Create a new Date object representing November 1, 1989
dt = new Date(1989, 10, 1);
// Output the seconds component of the date November 1, 1989
console.log(seconds_with_leading_zeros(dt));
Output:
India Standard Time India Standard Time
Explanation:
In the exercise above,
- The code defines a function named "seconds_with_leading_zeros()" that takes a Date object "dt" as input.
- Inside the seconds_with_leading_zeros function:
- It creates a new Date object representing the current date and time using 'new Date()'.
- It converts the current date object to a string representation using 'new Date().toString()'.
- It uses a regular expression (/\((.*)\)/) to extract the portion of the string enclosed in parentheses, which typically represents the timezone offset.
- Finally, it returns the extracted portion, which should contain the seconds component of the current date and time.
- After defining the function, the code creates a new Date object "dt" representing the current date and time using 'new Date()'. It then calls the "seconds_with_leading_zeros()" function with this Date object and outputs the result (the seconds component of the current date and time) to the console.
- Next, the code creates another new Date object "dt" representing November 1, 1989, using 'new Date(1989, 10, 1)'. It again calls the "seconds_with_leading_zeros()" function with this Date object and outputs the result to the console.
Flowchart:
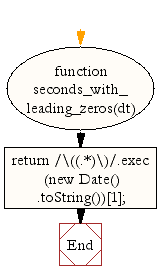
Live Demo:
See the Pen JavaScript - Get Timezone-date-ex-37 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that retrieves the timezone name from a Date object's string representation.
- Write a JavaScript function that uses Intl.DateTimeFormat to extract and return the full timezone name.
- Write a JavaScript function that converts a Date to a locale string and parses out the timezone information.
- Write a JavaScript function that handles cases where the timezone is not available and returns a default value.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get seconds with leading zeros (00 through 59).
Next: Write a JavaScript function to find whether or not the date is in daylights savings time.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.