JavaScript: Get seconds with leading zeros
JavaScript Datetime: Exercise-36 with Soutilon
Write a JavaScript function to get seconds with leading zeros (00 through 59).
Test Data:
dt = new Date();
console.log(seconds_with_leading_zeros(dt));
"India Standard Time"
Sample Solution:
JavaScript Code:
// Define a function seconds_with_leading_zeros that takes a Date object dt as input
function seconds_with_leading_zeros(dt)
{
// Check if the minutes component of the given date (dt.getMinutes()) is less than 10
// If it is less than 10, concatenate '0' to the minutes, else return an empty string
return (dt.getMinutes() < 10 ? '0' : '') + dt.getMinutes();
}
// Create a new Date object representing the current date and time
dt = new Date();
// Output the minutes component of the current date with leading zeros (if necessary)
console.log(seconds_with_leading_zeros(dt));
// Create a new Date object representing November 1, 1989
dt = new Date(1989, 10, 1);
// Output the minutes component of the date November 1, 1989 with leading zeros (if necessary)
console.log(seconds_with_leading_zeros(dt));
Output:
47 00
Explanation:
In the exercise above,
- The code defines a function named "seconds_with_leading_zeros()" that takes a Date object "dt" as input.
- Inside the seconds_with_leading_zeros function:
- It checks if the minutes component of the given date (dt.getMinutes()) is less than 10.
- If the minutes are less than 10, it concatenates a leading '0' to the minutes to ensure a consistent two-digit format.
- If the minutes are 10 or greater, it returns the minutes as is, without leading zeros.
- After defining the function, the code creates a new Date object "dt" representing the current date and time using 'new Date()'. It then calls the "seconds_with_leading_zeros()" function with this Date object and outputs the result (minutes with leading zeros, if necessary) to the console.
- Next, the code creates another new Date object "dt" representing November 1, 1989, using new Date(1989, 10, 1). It again calls the "seconds_with_leading_zeros()" function with this Date object and outputs the result to the console.
Flowchart:
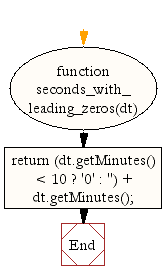
Live Demo:
See the Pen JavaScript - Get seconds with leading zeros-date-ex-36 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get minutes with leading zeros (00 to 59).
Next: Write a JavaScript function to get Timezone.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-date-exercise-36.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics