JavaScript: Get minutes with leading zeros
JavaScript Datetime: Exercise-35 with Solution
Minutes Leading Zeros
Write a JavaScript function to get minutes with leading zeros (00 to 59).
Test Data:
dt = new Date(1989, 10, 1);
console.log(minutes_with_leading_zeros(dt));
"00"
Sample Solution:
JavaScript Code:
// Define a JavaScript function called minutes_with_leading_zeros with parameter dt (date)
function minutes_with_leading_zeros(dt)
{
// Check if the minutes value of the provided date is less than 10
// If true, add a leading '0' to the minutes value; otherwise, return the minutes value as is
return (dt.getMinutes() < 10 ? '0' : '') + dt.getMinutes();
}
// Create a new Date object representing the current date
dt = new Date();
// Output the minutes value with leading zeroes for the current date
console.log(minutes_with_leading_zeros(dt));
// Create a new Date object representing November 1, 1989
dt = new Date(1989, 10, 1);
// Output the minutes value with leading zeroes for November 1, 1989
console.log(minutes_with_leading_zeros(dt));
Output:
33 00
Explanation:
In the exercise above,
- The code defines a JavaScript function named "minutes_with_leading_zeros()" with one parameter "dt", representing a Date object.
- Inside the "minutes_with_leading_zeros()" function:
- It checks if the minutes value of the provided Date object "dt" is less than 10.
- If the minutes value is less than 10, it adds a leading '0' to the minutes value using a ternary operator (<condition> ? <expression_if_true> : <expression_if_false>).
- If the minutes value is 10 or greater, it returns the minutes value as is.
- The code then demonstrates the usage of the "minutes_with_leading_zeros()" function:
- It creates a new Date object "dt" representing the current date using 'new Date()'.
- It calculates and outputs the minutes value with leading zeroes for the current date by calling the "minutes_with_leading_zeros()" function with "dt" and logging the result to the console.
- It creates another new Date object "dt" representing November 1, 1989, using 'new Date(1989, 10, 1)'.
- It calculates and outputs the minutes value with leading zeroes for November 1, 1989, by calling the "minutes_with_leading_zeros()" function with "dt" and logging the result to the console.
Flowchart:
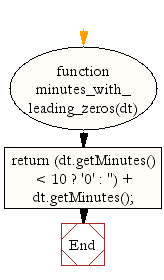
Live Demo:
See the Pen JavaScript - Get minutes with leading zeros-date-ex-35 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that returns the minutes of a Date object as a two-digit string using padStart().
- Write a JavaScript function that checks if the minutes are less than 10 and prepends a zero if necessary.
- Write a JavaScript function that extracts minutes from a date string and formats them with leading zeros.
- Write a JavaScript function that validates the minute value and always returns it in "MM" format.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get 24-hour format of an hour without leading zeros.
Next: Write a JavaScript function to get seconds with leading zeros (00 through 59).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics