JavaScript: Get 24-hour format of an hour without leading zeros
JavaScript Datetime: Exercise-34 with Solution
24-Hour Format
Write a JavaScript function to get the 24-hour format of an hour without leading zeros.
Test Data :
dt = new Date(1989, 10, 1);
console.log(hours_without_zeroes(dt));
0
Sample Solution:
JavaScript Code:
// Define a function named 'hours_without_zeroes' that takes a Date object 'dt' as input
function hours_without_zeroes(dt)
{
// Return the hour component of the given date, without leading zeroes
return dt.getHours();
}
// Create a new Date object representing the current date and time
dt = new Date();
// Print the hour component of the current date, without leading zeroes
console.log(hours_without_zeroes(dt));
// Create a new Date object representing a specific date (November 1, 1989)
dt = new Date(1989, 10, 1);
// Print the hour component of the specific date, without leading zeroes
console.log(hours_without_zeroes(dt));
Output:
15 0
Explanation:
The above JavaScript code defines a function named "hours_without_zeroes()" that takes a "Date" object as input and returns the hour component of the date without leading zeroes. It then creates two "Date" objects - one representing the current date and time, and another representing November 1, 1989. It prints the hour component of each date object using the "hours_without_zeroes()" function, demonstrating how it works.
Flowchart:
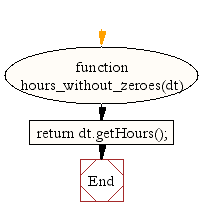
Live Demo:
See the Pen JavaScript - Get 24-hour format of an hour without leading zeros-date-ex-34 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that extracts the hour from a Date object and returns it in 24-hour format as a number.
- Write a JavaScript function that converts a 12-hour time string with AM/PM into 24-hour time without leading zeros.
- Write a JavaScript function that validates a Date object and outputs its hour in 24-hour format using getHours().
- Write a JavaScript function that processes user input in 12-hour format and returns the equivalent 24-hour number.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get 12-hour format of an hour with leading zeros.
Next: Write a JavaScript function to get minutes with leading zeros (00 to 59).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.