JavaScript: Get 12-hour format of an hour with leading zeros
JavaScript Datetime: Exercise-33 with Solution
12-Hour Format
Write a JavaScript function to get the 12-hour format of an hour with leading zeros.
Test Data:
dt = new Date(1989, 10, 1);
console.log(hours_with_zeroes(dt));
"12"
Sample Solution:
JavaScript Code:
// Define a JavaScript function called hours_with_zeroes with parameter dt (date)
function hours_with_zeroes(dt)
{
// Calculate the hours with leading zeroes based on the provided date
// If the hour value is less than 12, it takes the modulus with 12, otherwise it takes 12
// It then checks if the result is less than 10; if true, it adds a leading '0', otherwise it returns the result
return ((dt.getHours() % 12 || 12) < 10 ? '0' : '') + (dt.getHours() % 12 || 12);
}
// Create a new Date object representing the current date
dt = new Date();
// Output the hours with leading zeroes for the current date
console.log(hours_with_zeroes(dt));
// Create a new Date object representing November 1, 1989
dt = new Date(1989, 10, 1);
// Output the hours with leading zeroes for November 1, 1989
console.log(hours_with_zeroes(dt));
Output:
03 12
Explanation:
In the exercise above,
- The code defines a JavaScript function named "hours_with_zeroes()" with one parameter 'dt', representing a Date object.
- Inside the hours_with_zeroes function:
- It calculates the hours with leading zeroes based on the provided Date object "dt".
- It calculates the hour value by using "getHours()" method of the Date object.
- If the hour value is less than 12, it takes the modulus with 12; otherwise, it takes 12.
- It then checks if the resulting hour value is less than 10; if true, it adds a leading '0' to ensure a two-digit format, otherwise it returns the hour value as is.
- The code then demonstrates the usage of the "hours_with_zeroes()" function:
- It creates a new Date object "dt" representing the current date using 'new Date()'.
- It calculates and outputs the hours with leading zeroes for the current date by calling the "hours_with_zeroes()" function with 'dt' and logging the result to the console.
- It creates another new Date object "dt" representing November 1, 1989, using 'new Date(1989, 10, 1)'.
- It calculates and outputs the hours with leading zeroes for November 1, 1989, by calling the "hours_with_zeroes()" function with 'dt' and logging the result to the console.
Flowchart:
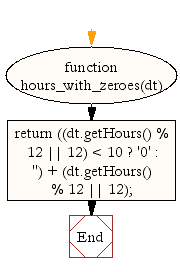
Live Demo:
See the Pen JavaScript - Get 12-hour format of an hour with leading zeros-date-ex-33 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to swatch Internet time (000 through 999).
Next: Write a JavaScript function to get 24-hour format of an hour without leading zeros.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics