JavaScript: Get the number of days in a month
JavaScript Datetime: Exercise-3 with Solution
Write a JavaScript function to get the number of days in a month.
Test Data:
console.log(getDaysInMonth(1, 2012));
console.log(getDaysInMonth(2, 2012));
console.log(getDaysInMonth(9, 2012));
console.log(getDaysInMonth(12, 2012));
Output :
31
29
30
31
Sample Solution:
JavaScript Code:
// Define a JavaScript function called getDaysInMonth with parameters month and year
var getDaysInMonth = function(month, year) {
// Get the number of days in the specified month and year
return new Date(year, month, 0).getDate();
// Here January is 0 based
// return new Date(year, month+1, 0).getDate();
};
// Output the number of days in January 2012
console.log(getDaysInMonth(1, 2012));
// Output the number of days in February 2012
console.log(getDaysInMonth(2, 2012));
// Output the number of days in September 2012
console.log(getDaysInMonth(9, 2012));
// Output the number of days in December 2012
console.log(getDaysInMonth(12, 2012));
Output:
31 29 30 31
Explanation:
In the exercise above -
- The code defines a JavaScript function named "getDaysInMonth()" with two parameters: 'month' and 'year'. This function is intended to calculate the number of days in a specific month of a given year.
- Inside the function:
- It creates a new Date object using the provided 'year' and 'month' parameters. The 'month' parameter is 1-based, where January is represented by 1.
- By setting the day parameter to 0 in the Date constructor, it gets the last day of the previous month, effectively representing the last day of the specified month.
- It then calls .getDate() on this Date object to retrieve the day component, which corresponds to the total number of days in the specified month.
- The function returns the calculated number of days.
- The code then provides several test cases using console.log() to output the results of calling the "getDaysInMonth()" function with different month and year combinations:
- January 2012
- February 2012 (leap year)
- September 2012
- December 2012
Flowchart:
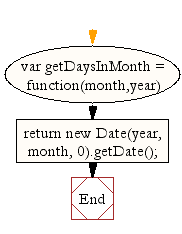
Live Demo:
See the Pen JavaScript - Get the number of days in a month-date-ex- 3 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get the current date.
Next: Write a JavaScript function to get the month name from a particular date.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-date-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics