JavaScript: Get a numeric representation of a month
JavaScript Datetime: Exercise-26 with Solution
Numeric Month
Write a JavaScript function to get a numeric representation of a month, with leading zeros (01 through 12).
Test Data:
dt = new Date(2015, 10, 1);
console.log(numeric_month(dt));
"11"
Sample Solution:
JavaScript Code:
// Define a JavaScript function called numeric_month with parameter dt (date)
function numeric_month(dt)
{
// Return the month in numeric format, ensuring that months below October are prefixed with '0'
return (dt.getMonth() < 9 ? '0' : '') + (dt.getMonth() + 1);
}
// Create a new Date object representing the current date
dt = new Date();
// Output the numeric representation of the month for the current date
console.log(numeric_month(dt));
// Create a new Date object representing November 1, 2015
dt = new Date(2015, 10, 1);
// Output the numeric representation of the month for November 1, 2015
console.log(numeric_month(dt));
Output:
06 11
Explanation:
In the exercise above,
- The code defines a JavaScript function named "numeric_month()" with one parameter 'dt', representing a Date object.
- Inside the numeric_month function:
- It retrieves the month index from the provided Date object "dt" using the "getMonth()" method. Note that the month index is zero-based (0 for January, 1 for February, ..., 11 for December).
- It checks if the month index is less than 9 (indicating October or earlier) to determine whether the month needs a leading zero.
- It returns the numeric representation of the month, ensuring that months below October are prefixed with '0' to maintain a two-digit format.
- The code then demonstrates the usage of the "numeric_month()" function:
- It creates a new Date object "dt" representing the current date using 'new Date()'.
- It outputs the numeric representation of the month for the current date by calling the "numeric_month()" function with 'dt' and logging the result to the console.
- It creates another new Date object 'dt' representing November 1, 2015, using 'new Date(2015, 10, 1)'.
- It outputs the numeric representation of the month for November 1, 2015, by calling the "numeric_month()" function with 'dt' and logging the result to the console.
Flowchart:
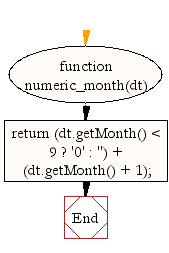
Live Demo:
See the Pen JavaScript - Get a numeric representation of a month-date-ex-26 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that returns the numeric month from a Date object as a two-digit string with a leading zero.
- Write a JavaScript function that converts the month index to a numeric representation and pads it to two digits.
- Write a JavaScript function that formats a Date’s month number using String.padStart() for consistency.
- Write a JavaScript function that validates the Date input and returns the month as both a number and a formatted string.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get a full textual representation of a month, such as January or June.
Next: Write a JavaScript function to get a short textual representation of a month, three letters (Jan through Dec).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.