JavaScript: Get ISO-8601 week number of year, weeks starting on Monday
JavaScript Datetime: Exercise-24 with Solution
ISO Week Number
Write a JavaScript function for obtaining ISO-8601 week numbers for weeks that begin on Monday.
Example : 42 (the 42nd week in the year)
Test Data :
dt = new Date(2015, 10, 1);
console.log(ISO8601_week_no(dt));
44
Sample Solution:
JavaScript Code:
// Define a JavaScript function called ISO8601_week_no with parameter dt (date)
function ISO8601_week_no(dt)
{
// Create a copy of the provided date object to avoid modifying the original
var tdt = new Date(dt.valueOf());
// Calculate the day number (0 for Sunday, 1 for Monday, ..., 6 for Saturday) and adjust it to have Monday as the first day of the week
var dayn = (dt.getDay() + 6) % 7;
// Adjust the copied date object to represent the Thursday of the current week
tdt.setDate(tdt.getDate() - dayn + 3);
// Store the value of the first Thursday of the year
var firstThursday = tdt.valueOf();
// Set the copied date object to January 1st of the current year
tdt.setMonth(0, 1);
// If January 1st is not a Thursday, find the date of the first Thursday of the year
if (tdt.getDay() !== 4)
{
tdt.setMonth(0, 1 + ((4 - tdt.getDay()) + 7) % 7);
}
// Calculate the ISO 8601 week number based on the difference between the value of the first Thursday and the current Thursday, divided by the number of milliseconds in a week
return 1 + Math.ceil((firstThursday - tdt) / 604800000);
}
// Create a new Date object representing the current date
dt = new Date();
// Output the ISO 8601 week number for the current date
console.log(ISO8601_week_no(dt));
// Create a new Date object representing November 1, 2015
dt = new Date(2015, 10, 1);
// Output the ISO 8601 week number for November 1, 2015
console.log(ISO8601_week_no(dt));
Output:
25 44
Explanation:
In the exercise above,
- The code defines a JavaScript function named "ISO8601_week_no()" with one parameter 'dt', representing a Date object.
- Inside the ISO8601_week_no function:
- It creates a copy of the provided date object to avoid modifying the original.
- It calculates the day number (0 for Sunday, 1 for Monday, ..., 6 for Saturday) and adjusts it to have Monday as the first day of the week.
- It adjusts the copied date object to represent Thursday of the current week.
- It stores the value of the first Thursday of the year.
- It sets the copied date object to January 1st of the current year.
- If January 1st is not a Thursday, it finds the date of the first Thursday of the year.
- It calculates the ISO 8601 week number based on the difference between the value of the first Thursday and the current Thursday, divided by the number of milliseconds in a week.
- The code then demonstrates the usage of the ISO8601_week_no function:
- It creates a new Date object "dt" representing the current date.
- It outputs the ISO 8601 week number for the current date by calling the "ISO8601_week_no()" function with "dt" and logging the result to the console.
- It creates another new Date object "dt" representing November 1, 2015.
- It outputs the ISO 8601 week number for November 1, 2015 by calling the "ISO8601_week_no()" function with "dt" and logging the result to the console.
Flowchart:
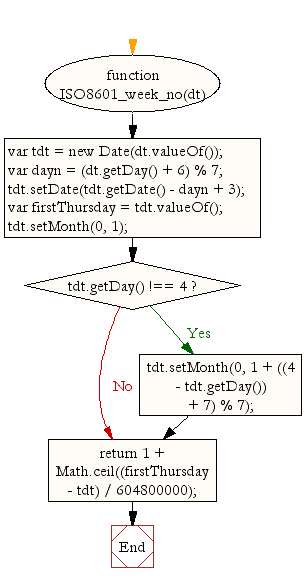
Live Demo:
See the Pen JavaScript - Get ISO-8601 week number of year, weeks starting on Monday-date-ex-24 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that computes the ISO week number for a given Date object using the ISO-8601 standard.
- Write a JavaScript function that handles edge cases at the beginning and end of the year when calculating the ISO week number.
- Write a JavaScript function that converts a Date to its corresponding week number and returns it as a number.
- Write a JavaScript function that validates a Date object and computes the week number with appropriate error handling.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get English ordinal suffix for the day of the month, 2 characters (st, nd, rd or th.).
Next: Write a JavaScript function to get a full textual representation of a month, such as January or June.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.