JavaScript: Get English ordinal suffix for the day of the month
JavaScript Datetime: Exercise-23 with Solution
Day Ordinal Suffix
Write a JavaScript function to get the English ordinal suffix for the day of the month, 2 characters (st, nd, rd, or th).
Test Data :
dt= new Date();
console.log(english_ordinal_suffix(dt));
"23rd"
dt = new Date(2015, 10, 1);
console.log(english_ordinal_suffix(dt));
"1st"
Sample Solution:
JavaScript Code:
// Define a JavaScript function called english_ordinal_suffix with parameter dt (date)
function english_ordinal_suffix(dt)
{
// Return the day of the month with its ordinal suffix (e.g., 1st, 2nd, 3rd, 4th)
return dt.getDate() +
(dt.getDate() % 10 == 1 && dt.getDate() != 11 ? 'st' :
(dt.getDate() % 10 == 2 && dt.getDate() != 12 ? 'nd' :
(dt.getDate() % 10 == 3 && dt.getDate() != 13 ? 'rd' : 'th')));
}
// Create a new Date object representing the current date
dt = new Date();
// Output the day of the month with its ordinal suffix for the current date
console.log(english_ordinal_suffix(dt));
// Create a new Date object representing November 1, 2015
dt = new Date(2015, 10, 1);
// Output the day of the month with its ordinal suffix for November 1, 2015
console.log(english_ordinal_suffix(dt));
Output:
20th 1st
Explanation:
In the exercise above,
- The code defines a JavaScript function named "english_ordinal_suffix()" with one parameter 'dt', representing a Date object.
- Inside the english_ordinal_suffix function:
- It retrieves the day of the month from the provided Date object "dt" using the "getDate()" method.
- It checks the remainder when dividing the day of the month by 10 to determine the suffix ('st', 'nd', 'rd', 'th') to append.
- It also checks for exceptions where the suffix differs (e.g., 1st, 2nd, 3rd, but then 'th' for all other cases).
- It constructs and returns the day of the month along with its appropriate ordinal suffix.
- The code then demonstrates the usage of the "english_ordinal_suffix()" function:
- It creates a new Date object "dt" representing the current date (new Date()).
- It outputs the day of the month with its ordinal suffix for the current date by calling the "english_ordinal_suffix()" function with "dt" and logging the result to the console.
- It creates another new Date object "dt" representing November 1, 2015 (new Date(2015, 10, 1)).
- It outputs the day of the month with its ordinal suffix for November 1, 2015 by calling the "english_ordinal_suffix()" function with "dt" and logging the result to the console.
Flowchart:
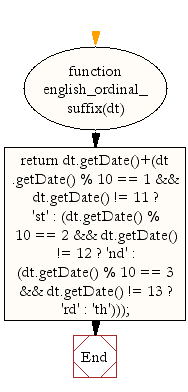
Live Demo:
See the Pen JavaScript - Get English ordinal suffix for the day of the month-date-ex-23 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that appends the correct English ordinal suffix to a numeric day of the month.
- Write a JavaScript function that takes a Date object, extracts the day, and returns it with the proper ordinal suffix.
- Write a JavaScript function that correctly handles special cases (11th, 12th, 13th) when assigning ordinal suffixes.
- Write a JavaScript function that converts a numeric day to a string with its ordinal suffix using a series of conditionals.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get a full textual representation of the day of the week (Sunday through Saturday).
Next: Write a JavaScript function to get ISO-8601 week number of year, weeks starting on Monday.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.