JavaScript: Get the day of the month, 2 digits with leading zeros
JavaScript Datetime: Exercise-19 with Solution
Day of Month (2 Digits)
Write a JavaScript function to get the day of the month, 2 digits with leading zeros.
Test Data :
d= new Date(2015, 10, 1);
console.log(day_of_the_month(d));
19
01
Sample Solution:
JavaScript Code:
// Define a JavaScript function called day_of_the_month with parameter d (date)
function day_of_the_month(d)
{
// Return the day of the month as a string
// If the day is less than 10, prepend '0' to the day to ensure two-digit formatting
return (d.getDate() < 10 ? '0' : '') + d.getDate();
}
// Create a new Date object representing the current date
d= new Date();
// Output the day of the month for the current date
console.log(day_of_the_month(d));
// Create a new Date object representing November 1, 2015
d= new Date(2015, 10, 1);
// Output the day of the month for November 1, 2015
console.log(day_of_the_month(d));
Output:
19 01
Explanation:
In the exercise above,
- The code defines a JavaScript function named "day_of_the_month()" with one parameter 'd', which represents a Date object.
- Inside the function:
- It extracts the day of the month from the provided Date object using the "getDate()" method.
- It checks if the extracted day is less than 10.
- If the day is less than 10, it prepends '0' to the day to ensure two-digit formatting.
- It returns the day of the month as a string.
- The code then demonstrates the usage of the "day_of_the_month()" function:
- It creates a new Date object representing the current date (new Date()).
- It outputs the day of the month for the current date using console.log().
- It creates a new Date object representing November 1, 2015 (new Date(2015, 10, 1)).
- It outputs the day of the month for November 1, 2015 using console.log().
Flowchart:
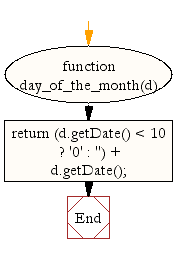
Live Demo:
See the Pen JavaScript - Get the day of the month, 2 digits with leading zeros-date-ex-19 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to calculate age.
Next: Write a JavaScript function to get a textual representation of a day (three letters, Mon through Sun).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics