JavaScript: Get the amount of days of a year
JavaScript Datetime: Exercise-14 with Solution
Days in Year
Write a JavaScript function to get the number of days in a year.
Test Data :
console.log(days_of_a_year(2015));
365
console.log(days_of_a_year(2016));
366
Sample Solution:
JavaScript Code:
// Define a JavaScript function called days_of_a_year with parameter year
function days_of_a_year(year)
{
// Return 366 if the given year is a leap year, otherwise return 365
return isLeapYear(year) ? 366 : 365;
}
// Define a JavaScript function called isLeapYear with parameter year
function isLeapYear(year) {
// Return true if the given year is divisible by 400 or divisible by 4 but not divisible by 100
return year % 400 === 0 || (year % 100 !== 0 && year % 4 === 0);
}
// Output the number of days in the year 2015
console.log(days_of_a_year(2015));
// Output the number of days in the year 2016
console.log(days_of_a_year(2016));
Output:
365 366
Explanation:
In the exercise above,
- The code defines a JavaScript function named "days_of_a_year()" with one parameter 'year', which represents the year for which we want to calculate the number of days.
- Inside the "days_of_a_year()" function:
- It returns the result of calling the "isLeapYear()" function with the given year.
- If the given year is a leap year (as determined by the "isLeapYear()" function), it returns 366, indicating that the year has 366 days.
- If the given year is not a leap year, it returns 365, indicating that the year has 365 days.
- The "isLeapYear()" function checks whether a given year is a leap year.
- It returns 'true' if the year is divisible by 400 or if the year is divisible by 4 but not divisible by 100, indicating that it's a leap year.
- Otherwise, it returns 'false'.
Flowchart:
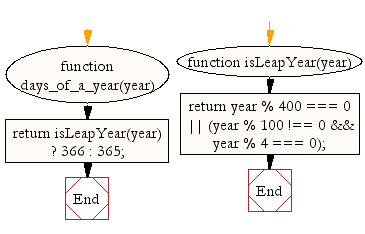
Live Demo:
See the Pen JavaScript - Get the amount of days of a year-date-ex- 14 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that determines if a given year is a leap year and returns 366 or 365 accordingly.
- Write a JavaScript function that calculates the number of days in a year by comparing January 1st of the year to January 1st of the next year.
- Write a JavaScript function that accepts a year and uses leap year logic to compute the correct number of days.
- Write a JavaScript function that validates the input year and handles non-numeric values gracefully.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function that will return the number of minutes in hours and minutes.
Next: Write a JavaScript function to get the quarter (1 to 4) of the year.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.