JavaScript: Minimum date from an array of dates
JavaScript Datetime: Exercise-12 with Solution
Write a JavaScript function to get the minimum date from an array of dates.
Test Data:
console.log(min_date(['2015/02/01', '2015/02/02', '2015/01/03']));
Output :
"2015/01/03"
Sample Solution:
JavaScript Code:
// Define a JavaScript function called min_date with parameter all_dates
function min_date(all_dates) {
// Initialize variables to store the minimum date and its corresponding Date object
var min_dt = all_dates[0],
min_dtObj = new Date(all_dates[0]);
// Iterate through each date in the array using forEach method
all_dates.forEach(function(dt, index) {
// Convert the current date string to a Date object
var current_dtObj = new Date(dt);
// Compare the current Date object with the minimum Date object
if (current_dtObj < min_dtObj) {
// If the current Date object is less than the minimum Date object, update the minimum date
min_dt = dt;
min_dtObj = new Date(dt);
}
});
// Return the minimum date
return min_dt;
}
// Output the minimum date from the provided array of dates
console.log(min_date(['2015/02/01', '2015/02/02', '2015/01/03']));
Output:
2015/01/03
Explanation:
In the exercise above,
- The code defines a JavaScript function named "min_date()" with one parameter 'all_dates', representing an array of date strings.
- Inside the function:
- It initializes two variables: 'min_dt' to store the minimum date string found so far and 'min_dtObj' to store the corresponding Date object of the minimum date.
- It converts the first date string in the 'all_dates' array to a Date object and assigns it to 'min_dtObj'.
- It iterates through each date string in the 'all_dates' array using the "forEach()" method.
- Inside the loop:
- It converts the current date string to a Date object.
- It compares the current Date object with the minimum Date object (min_dtObj).
- If the current Date object is less than 'min_dtObj', it updates 'min_dt' and 'min_dtObj' to the current date string and Date object, respectively.
- After iterating through all date strings, it returns the minimum date ('min_dt').
- The code then demonstrates the "min_date()" function by calling it with an array of date strings ['2015/02/01', '2015/02/02', '2015/01/03'].
Flowchart:
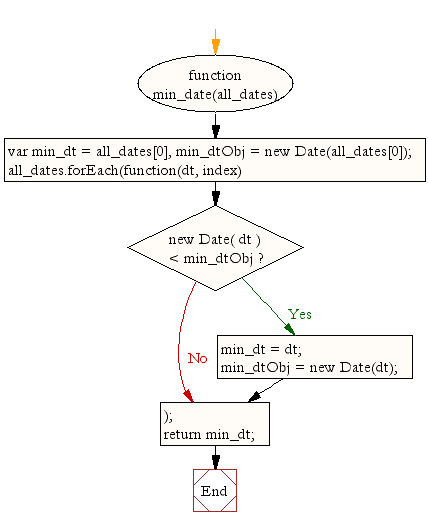
Live Demo:
See the Pen JavaScript - Minimum date from an array of dates-date-ex- 12 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get the maximum date from an array of dates.
Next: Write a JavaScript function that will return the number of minutes in hours and minutes.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-date-exercise-12.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics