JavaScript: Check whether an input is a date object or not
JavaScript Datetime: Exercise-1 with Solution
Check Date Object
Write a JavaScript function to check whether an 'input' is a date object or not.
Test Data:
console.log(is_date("October 13, 2014 11:13:00"));
console.log(is_date(new Date(86400000)));
console.log(is_date(new Date(99,5,24,11,33,30,0)));
console.log(is_date([1, 2, 4, 0]));
Output :
false
true
true
false
Sample Solution:
JavaScript Code:
// Check if the input is a date object
var is_date = function(input) {
// If the internal [[Class]] property of the input matches "[object Date]", return true
if (Object.prototype.toString.call(input) === "[object Date]")
return true;
// Otherwise, return false
return false;
};
// Test cases
console.log(is_date("October 13, 2014 11:13:00")); // false
console.log(is_date(new Date(86400000))); // true
console.log(is_date(new Date(99,5,24,11,33,30,0))); // true
console.log(is_date([1, 2, 4, 0])); // false
Output:
false true true false
Explanation:
The above code defines a JavaScript function named "is_date()" using the "var" keyword. This function takes one parameter 'input', which represents the value to be checked.
- Inside the function, it uses the Object.prototype.toString.call() method to determine the type of the input parameter. This method returns a string representation of the object's internal [[Class]] property.
- The function checks if the string representation matches "[object Date]", which is the string representation of a Date object's class.
- If the comparison matches, meaning the input is a Date object, the function returns 'true'.
- If the comparison fails, indicating that the input is not a Date object, the function returns 'false'.
Flowchart:
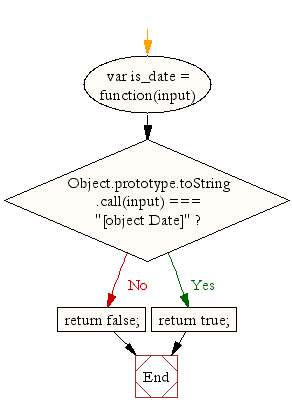
Live Demo:
See the Pen JavaScript - common-editor-exercises-date-ex- 1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that checks if a given input is a Date object using Array.prototype.toString.
- Write a JavaScript function that verifies a Date object by testing if getTime() returns a valid number.
- Write a JavaScript function that uses the instanceof operator to determine if the input is a Date.
- Write a JavaScript function that examines the input’s constructor name and returns true if it is "Date".
Improve this sample solution and post your code through Disqus.
Previous: Javascript date Exercises
Next: Write a JavaScript function to get the current date.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.