JavaScript: Find and print the first 5 happy numbers
JavaScript Conditional Statement and loops: Exercise-8 with Solution
Happy Numbers (First 5)
Happy Numbers:
According to Wikipedia a happy number is defined by the following process : "Starting with any positive integer, replace the number by the sum of the squares of its digits, and repeat the process until the number equals 1 (where it will stay), or it loops endlessly in a cycle which does not include 1. Those numbers for which this process ends in 1 are happy numbers, while those that do not end in 1 are unhappy numbers (or sad numbers)". Write a JavaScript program to find and print the first 5 happy numbers.
Visual Presentation:
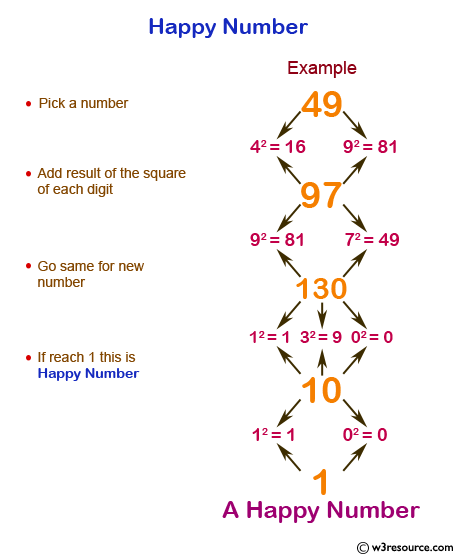
Sample Solution:
JavaScript Code:
// Function to check if a number is a happy number
function happy_number(num) {
var m, n;
var c = [];
// Continue loop until the number becomes 1 or enters a cycle
while (num !== 1 && c[num] !== true) {
c[num] = true;
m = 0;
// Calculate the sum of the squares of digits
while (num > 0) {
n = num % 10;
m += n * n;
num = (num - n) / 10;
}
num = m;
}
return (num === 1);
}
// Variables to track the count and current number
var cnt = 5;
var num = 1;
var f5 = '';
// Find the first 5 happy numbers
while (cnt-- > 0) {
while (!happy_number(num))
num++;
f5 = f5 + (num + ", ");
num++;
}
console.log('First 5 happy numbers are: ' + f5);
Output:
First 5 happy numbers are : 1, 7, 10, 13, 19,
Flowchart:
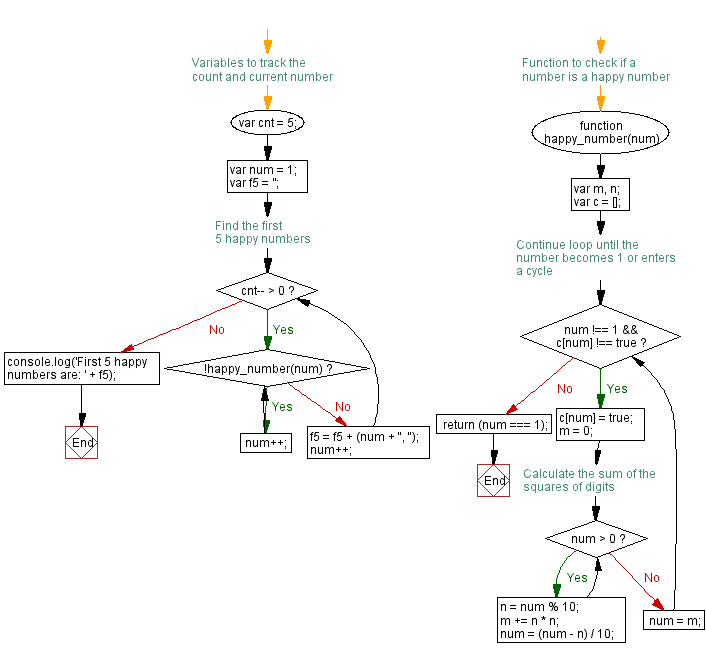
Live Demo:
See the Pen javascript-conditional-statements-and-loops-exercise-8 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that finds the first 5 happy numbers using a helper function to compute the sum of the squares of digits.
- Write a JavaScript function that identifies happy numbers by recursively computing digit square sums until 1 is reached.
- Write a JavaScript function that prints the first 5 happy numbers by iterating through natural numbers and checking for cycles.
- Write a JavaScript function that generates an array of the first 5 happy numbers while optimizing the check for repeated cycles.
Go to:
PREV : FizzBuzz.
NEXT : 3-Digit Armstrong Numbers.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.