JavaScript: Iterates the integers from 1 to 100
JavaScript Conditional Statement and loops: Exercise-7 with Solution
FizzBuzz
Write a JavaScript program that iterates integers from 1 to 100. But for multiples of three print "Fizz" instead of the number and for multiples of five print "Buzz". For numbers multiples of both three and five print "FizzBuzz".
Sample Solution:
JavaScript Code:
// Loop through numbers from 1 to 100
for (var i = 1; i <= 100; i++) {
// Check if the number is divisible by both 3 and 5
if (i % 3 === 0 && i % 5 === 0) {
console.log(i + " FizzBuzz");
}
// Check if the number is divisible by 3
else if (i % 3 === 0) {
console.log(i + " Fizz");
}
// Check if the number is divisible by 5
else if (i % 5 === 0) {
console.log(i + " Buzz");
}
// If none of the above conditions are met, print the number
else {
console.log(i);
}
}
Output:
1 2 3 Fizz 4 5 Buzz 6 Fizz 7 8 9 Fizz 10 Buzz -----
Flowchart:
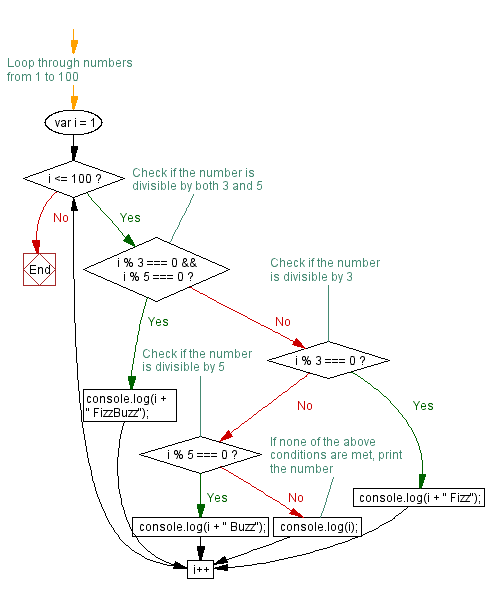
Live Demo:
See the Pen javascript-conditional-statements-and-loops-exercise-7 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that implements FizzBuzz for numbers 1 to 100 using nested ternary operators.
- Write a JavaScript function that generates an array of FizzBuzz outputs for numbers 1 to 100 and returns it.
- Write a JavaScript function that implements FizzBuzz recursively for numbers 1 to 100.
- Write a JavaScript function that outputs FizzBuzz results for 1 to 100 without using any conditional statements.
Go to:
PREV : Student Grades Calculation.
NEXT : Happy Numbers (First 5).
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.