JavaScript: For loop that will iterate from 0 to 15 to find even and odd numbers
JavaScript Conditional Statement and loops: Exercise-5 with Solution
Odd or Even Loop
Write a JavaScript for loop that iterates from 0 to 15. For each iteration, it checks if the current number is odd or even, and displays a message on the screen.
Sample Output:
"0 is even"
"1 is odd"
"2 is even"
----------
----------
Calculate a Even Number:
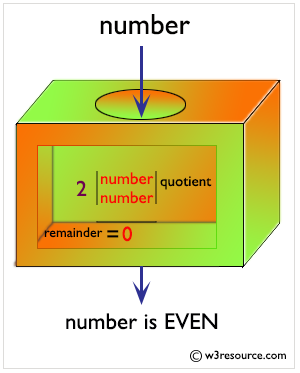
Even Numbers between 1 to 100:
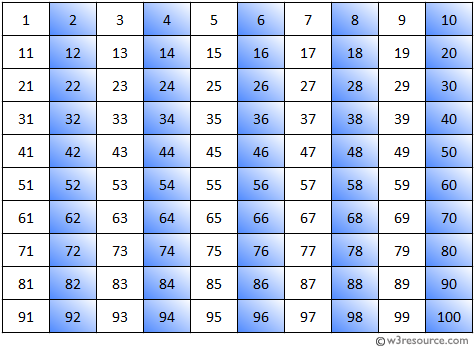
Calculate a Odd Number:
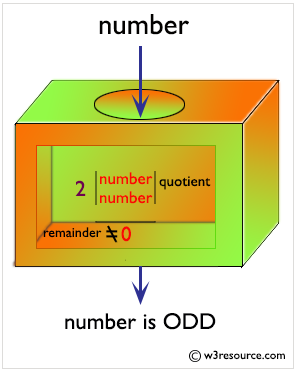
Odd Numbers between 1 to 100:
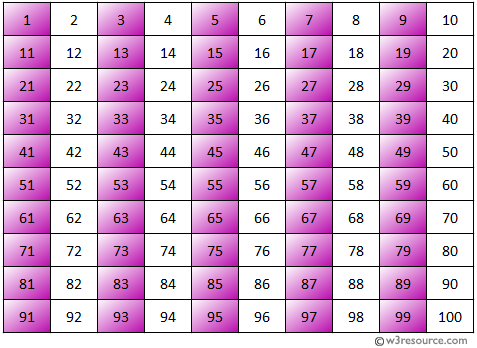
Sample Solution:
JavaScript Code:
// Using a for loop to iterate through numbers from 0 to 15
for (var x = 0; x <= 15; x++) {
// Checking if the current number is 0
if (x === 0) {
console.log(x + " is even");
}
// Checking if the current number is even
else if (x % 2 === 0) {
console.log(x + " is even");
}
// If the number is not 0 and not even, it is odd
else {
console.log(x + " is odd");
}
}
Output:
0 is even 1 is odd 2 is even 3 is odd 4 is even 5 is odd 6 is even 7 is odd 8 is even 9 is odd 10 is even 11 is odd 12 is even 13 is odd 14 is even 15 is odd
Flowchart:
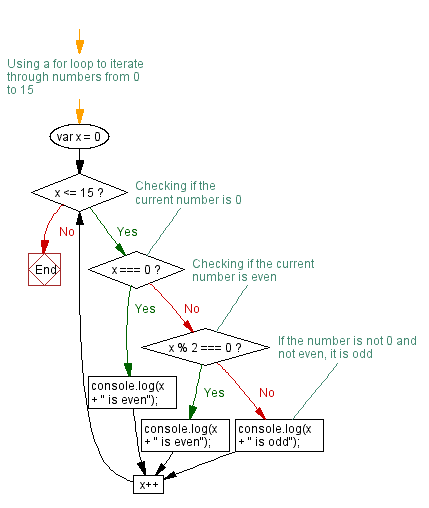
Live Demo:
See the Pen javascript-conditional-statements-and-loops-exercise-5 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that iterates from 0 to 15 and prints whether each number is odd or even using a ternary operator.
- Write a JavaScript function that determines odd or even status from 0 to 15 without using the modulo operator.
- Write a JavaScript function that checks numbers from 0 to 15 for odd or even status using bitwise operations.
- Write a JavaScript function that loops through 0 to 15, printing odd/even status while also identifying prime numbers.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript conditional statement to find the largest of five numbers.
Next: Write a JavaScript program which compute, the average marks of the following students Then, this average is used to determine the corresponding grade.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.