JavaScript: Find the largest of five numbers
JavaScript Conditional Statement and loops: Exercise-4 with Solution
Largest of Five Numbers
Write a JavaScript conditional statement to find the largest of five numbers. Display an alert box to show the results.
Sample numbers : -5, -2, -6, 0, -1
Output : 0
Visual Presentation:
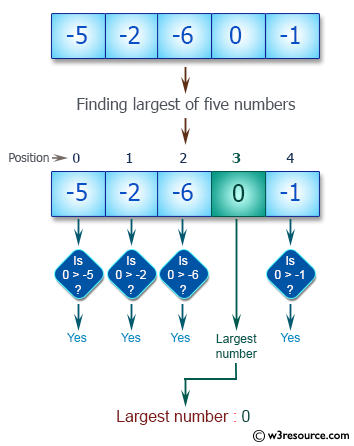
Sample Solution:
JavaScript Code:
// Assigning values to variables
var a = -5;
var b = -2;
var c = -6;
var d = 0;
var f = -1;
// Checking the conditions to determine the maximum value
if (a > b && a > c && a > d && a > f) {
console.log(a);
} else if (b > a && b > c && b > d && b > f) {
console.log(b);
} else if (c > a && c > b && c > d && c > f) {
console.log(c);
} else if (d > a && d > c && d > b && d > f) {
console.log(d);
} else {
console.log(f);
}
Output:
0
Flowchart:
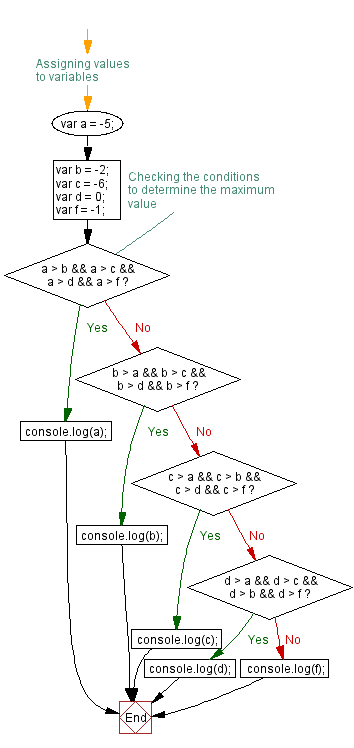
Live Demo:
See the Pen javascript-conditional-statements-and-loops-exercise-4 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript conditional statement to sort three numbers. Display an alert box to show the result.
Next: Write a JavaScript for loop that will iterate from 0 to 15.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics