JavaScript: How to sort three numbers
JavaScript Conditional Statement and loops: Exercise-3 with Solution
Sort Three Numbers
Write a JavaScript conditional statement to sort three numbers. Display an alert box to show the results.
Sample numbers : 0, -1, 4
Output : 4, 0, -1
Visual Presentation:
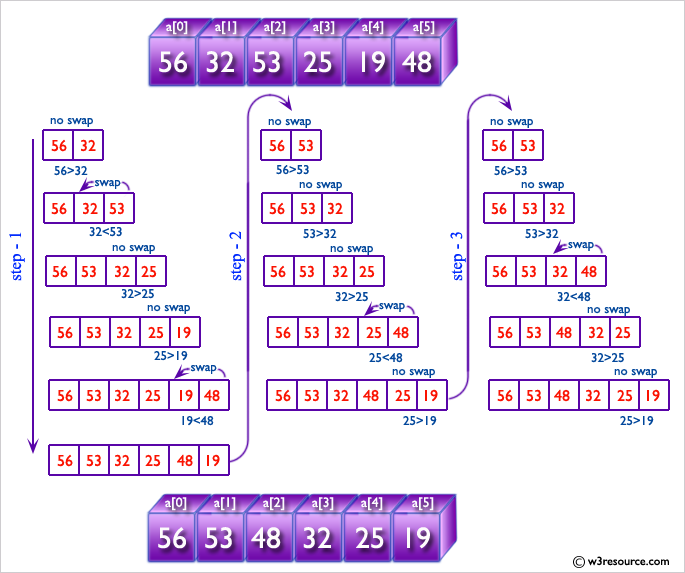
Sample Solution:
JavaScript Code:
// Assigning values to variables
var x = 0;
var y = -1;
var z = 4;
// Checking the conditions to determine the order of variables
if (x > y && x > z) {
if (y > z) {
console.log(x + ", " + y + ", " + z);
} else {
console.log(x + ", " + z + ", " + y);
}
} else if (y > x && y > z) {
if (x > z) {
console.log(y + ", " + x + ", " + z);
} else {
console.log(y + ", " + z + ", " + x);
}
} else if (z > x && z > y) {
if (x > y) {
console.log(z + ", " + x + ", " + y);
} else {
console.log(z + ", " + y + ", " + x);
}
}
Output:
4, 0, -1
Flowchart:
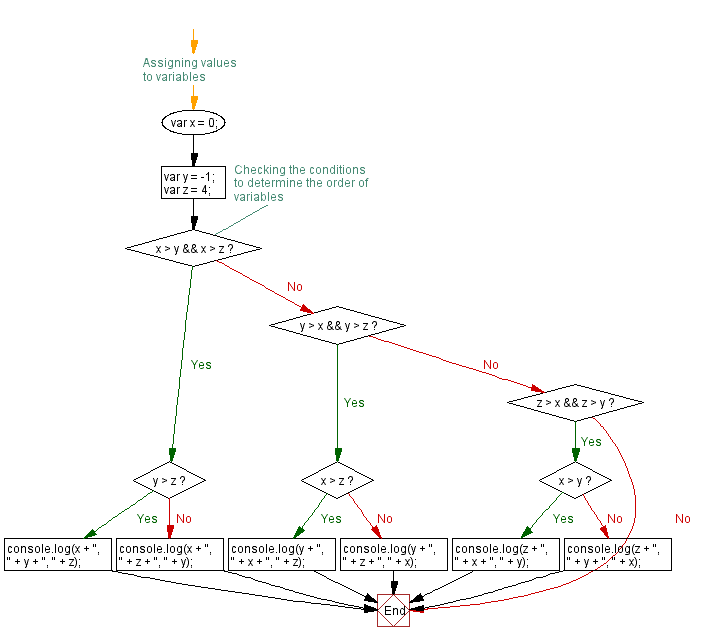
Live Demo:
See the Pen javascript-conditional-statements-and-loops-exercise-3 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that sorts three numbers in descending order using only arithmetic operations.
- Write a JavaScript function that sorts three integers without using any built-in sort methods or conditional (if-else) statements.
- Write a JavaScript function that identifies the median, maximum, and minimum of three numbers by comparing their differences.
- Write a JavaScript function that orders three numbers in ascending order by leveraging the properties of the absolute difference between them.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript conditional statement to find the sign of product of three numbers. Display an alert box with the specified sign.
Next: Write a JavaScript conditional statement to find the largest of five numbers. Display an alert box to show the result.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.