JavaScript: Compute the greatest common divisor (GCD) of two positive integers
JavaScript Conditional Statement and loops: Exercise-11 with Solution
GCD of Two Numbers
Write a JavaScript program to compute the greatest common divisor (GCD) of two positive integers.
Visual Presentation:
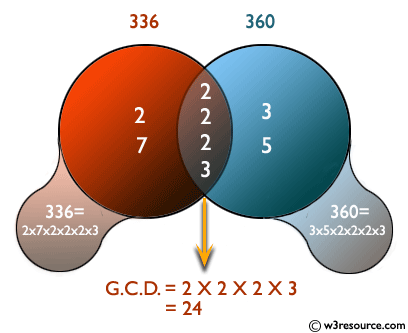
Sample Solution:
JavaScript Code:
// Variables to store the two numbers for which GCD is calculated
var a = 2154; // First number
var b = 458; // Second number
var gcd; // Variable to store the Greatest Common Divisor (GCD)
// Iterative loop to find GCD using Euclidean algorithm
while (a != b) {
// If 'a' is greater than 'b', subtract 'b' from 'a'
if (a > b) {
a = a - b;
} else {
// If 'b' is greater than 'a', subtract 'a' from 'b'
b = b - a;
}
}
// The GCD is stored in 'a'
gcd = a;
// Output the calculated GCD
console.log(gcd);
Output:
2
Flowchart:
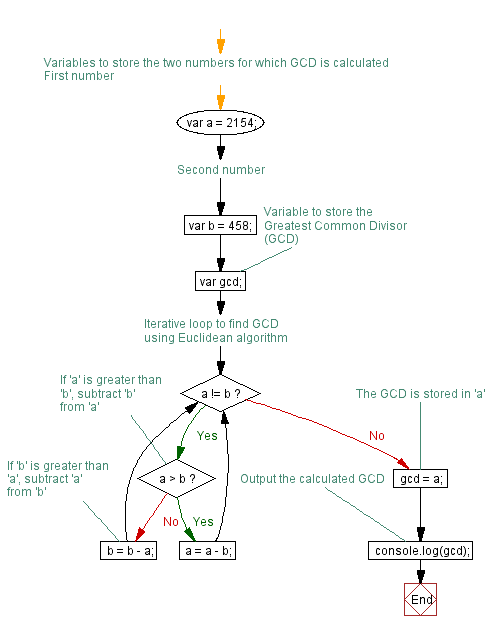
Live Demo:
See the Pen javascript-conditional-statements-and-loops-exercise-11 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that computes the greatest common divisor of two numbers using the iterative Euclidean algorithm.
- Write a JavaScript function that calculates the GCD of two numbers recursively while handling negative inputs.
- Write a JavaScript function that finds the GCD of two numbers without using the modulo operator.
- Write a JavaScript function that computes the GCD by using repeated subtraction instead of division.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to construct the following pattern, using a nested for loop.
Next: Write a JavaScript program to sum the multiples of 3 and 5 under 1000.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.