JavaScript: Accept two integers and display the larger
JavaScript Conditional Statement and loops: Exercise-1 with Solution
Largest of Two Integers
Write a JavaScript program that displays the largest integer among two integers.
Visual Presentation:
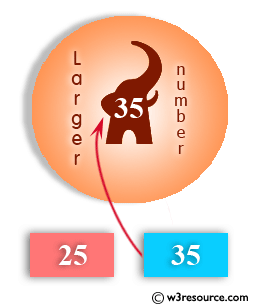
Sample Solution-1:
JavaScript Code:
// Declare variables to store input values
var num1, num2;
// Uncomment the following lines to take input from the user
// num1 = window.prompt("Input the First integer", "0");
// num2 = window.prompt("Input the second integer", "0");
// Compare the values and print the larger or equality message
if (parseInt(num1, 10) > parseInt(num2, 10)) {
console.log("The larger of " + num1 + " and " + num2 + " is " + num1 + ".");
} else if (parseInt(num2, 10) > parseInt(num1, 10)) {
console.log("The larger of " + num1 + " and " + num2 + " is " + num2 + ".");
} else {
console.log("The values " + num1 + " and " + num2 + " are equal.");
}
Output:
The values null and null are equal.
Flowchart:
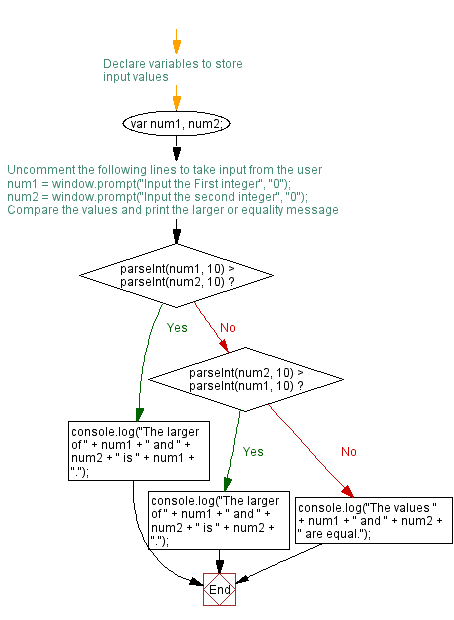
Live Demo:
See the Pen javascript-conditional-statements-and-loops-exercise-1 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Function to compare two numbers and return the larger one or a message for equality
function compareNumbers(num1, num2) {
if (num1 > num2) {
return "The larger of " + num1 + " and " + num2 + " is " + num1 + ".";
} else if (num2 > num1) {
return "The larger of " + num1 + " and " + num2 + " is " + num2 + ".";
} else {
return "The values " + num1 + " and " + num2 + " are equal.";
}
}
// Example usage
var result = compareNumbers(5, 8);
console.log(result);
result = compareNumbers(10, 5);
console.log(result);
result = compareNumbers(7, 7);
console.log(result);
Output:
The larger of 5 and 8 is 8. The larger of 10 and 5 is 10. The values 7 and 7 are equal.
Flowchart:
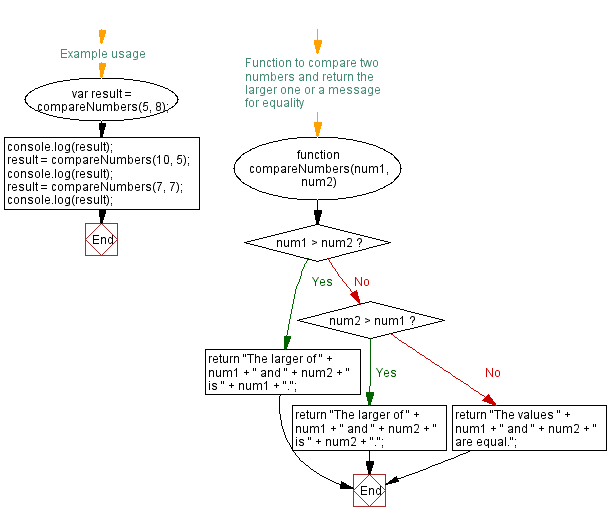
Contribute your code and comments through Disqus.
Previous: Javascript Conditional Statements and Loops Exercises
Next: Write a JavaScript conditional statement to find the sign of product of three numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics