JavaScript: Rearrange characters of a given string in such way that it will become equal to another given string
JavaScript Basic: Exercise-99 with Solution
Write a JavaScript program to check whether it is possible to rearrange the characters of a given string. This is in such a way that it will become equal to another given string.
Sample Solution:
JavaScript Code:
// Function to check if characters of str1 can be rearranged to form str2
function rearrangement_characters(str1, str2) {
// Splitting the strings into arrays of characters
var first_set = str1.split(''), // Array for characters of str1
second_set = str2.split(''), // Array for characters of str2
result = true; // Variable to store the result of rearrangement check
// Sorting the character arrays in ascending order
first_set.sort();
second_set.sort();
// Loop through the arrays to compare each character position
for (var i = 0; i < Math.max(first_set.length, second_set.length); i++) {
// Check if characters at the same position in both arrays are different
if (first_set[i] !== second_set[i]) {
result = false; // Set result to false if characters differ
}
}
return result; // Return the final result of rearrangement check
}
// Testing the function with sample strings
console.log(rearrangement_characters("xyz", "zyx")); // Example usage
console.log(rearrangement_characters("xyz", "zyp")); // Example usage
Output:
true false
Live Demo:
See the Pen javascript-basic-exercise-99 by w3resource (@w3resource) on CodePen.
Flowchart:
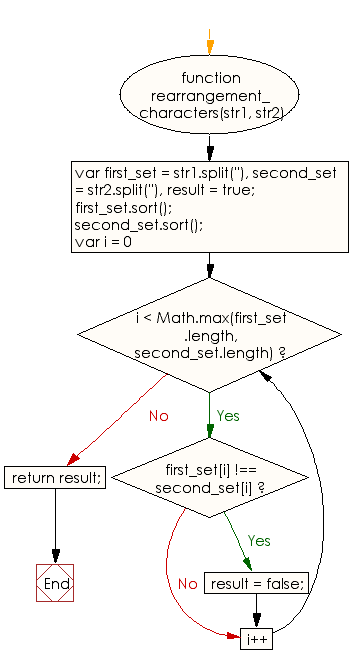
ES6 Version:
// Function to check if two strings can be rearranged to form each other
const rearrangement_characters = (str1, str2) => {
// Splitting both strings into arrays of characters
let first_set = str1.split(''); // Array from first string
let second_set = str2.split(''); // Array from second string
let result = true; // Initializing result as true
// Sorting both arrays
first_set.sort();
second_set.sort();
// Looping through the arrays to compare elements
for (let i = 0; i < Math.max(first_set.length, second_set.length); i++) {
if (first_set[i] !== second_set[i]) {
result = false; // If any character at the same index is different, set result to false
}
}
return result; // Return the result
}
console.log(rearrangement_characters("xyz", "zyx")); // Example usage
console.log(rearrangement_characters("xyz", "zyp")); // Example usage
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to switch case of the minimum possible number of letters to make a given string written in the upper case or in the lower case.
Next: JavaScript program to check if there is at least one element which occurs in two given sorted arrays of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/javascript-basic-exercise-99.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics