JavaScript: Switch case of the minimum possible number of letters to make a given string written in the upper case or in the lower case
JavaScript Basic: Exercise-98 with Solution
Change Case Minimally for Upper or Lower Case
Write a JavaScript program to change the case of the minimum number of letters to make a given string written in upper case or lower case.
Fox example "Write" will be write and "PHp" will be "PHP"
Sample Solution:
JavaScript Code:
// Function to change case based on the character count of upper and lower case letters
function change_case(new_str) {
var x = 0; // Variable to count the number of uppercase letters
var y = 0; // Variable to count the number of lowercase letters
// Loop through each character in the input string
for (var i = 0; i < new_str.length; i++) {
// Check if the current character is an uppercase letter using a regular expression
if (/[A-Z]/.test(new_str[i])) {
x++; // Increment the count of uppercase letters
} else {
y++; // Increment the count of lowercase letters
}
}
// Compare the counts and change the case of the input string accordingly
if (y > x) {
return new_str.toLowerCase(); // If lowercase count is greater, return string in lowercase
}
return new_str.toUpperCase(); // Otherwise, return string in uppercase
}
console.log(change_case("Write")); // Output the result of the function for the input "Write"
console.log(change_case("PHp")); // Output the result of the function for the input "PHp"
Output:
write PHP
Live Demo:
See the Pen javascript-basic-exercise-98 by w3resource (@w3resource) on CodePen.
Flowchart:
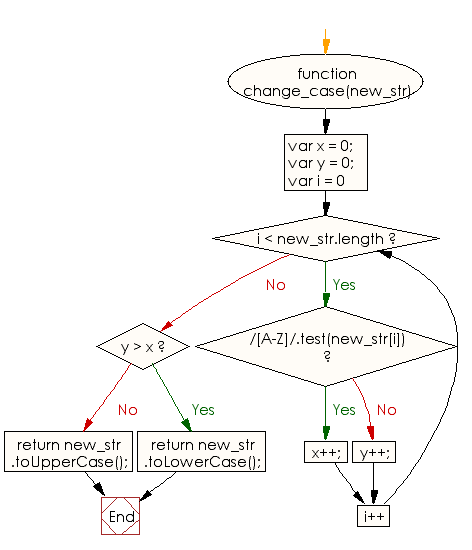
ES6 Version:
// Function to change the case of the string based on character counts
const change_case = (new_str) => {
let x = 0; // Variable to count uppercase characters
let y = 0; // Variable to count lowercase characters
// Loop through each character of the string
for (let i = 0; i < new_str.length; i++) {
if (/[A-Z]/.test(new_str[i])) {
x++; // Increment the count of uppercase characters
} else {
y++; // Increment the count of lowercase characters
}
}
// If the count of lowercase characters is greater, convert the string to lowercase; otherwise, convert to uppercase
if (y > x) {
return new_str.toLowerCase();
}
return new_str.toUpperCase();
}
console.log(change_case("Write")); // Example usage
console.log(change_case("PHp")); // Example usage
For more Practice: Solve these Related Problems:
- Write a JavaScript program that analyzes a string and converts it entirely to upper or lower case based on which option requires fewer character changes.
- Write a JavaScript function that computes the number of changes needed to convert a string to all uppercase versus all lowercase, then applies the minimal change option.
- Write a JavaScript program that transforms a string to uniform case (upper or lower) by altering the minimal number of characters, and returns the converted string.
Go to:
PREV : Shortest String to Convert into Palindrome.
NEXT : Check if String Can Rearrange to Match Another.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.