JavaScript: Find the shortest possible string which can create a string to make it a palindrome by adding characters to the end of it
JavaScript Basic: Exercise-97 with Solution
Shortest String to Convert into Palindrome
Write a JavaScript program to find the shortest possible string. This can be converted into a string and converted into a palindrome by adding characters to the end of it.
Sample Solution:
JavaScript Code:
// Function to build a palindrome from a given string
function build_Palindrome(new_str) {
var flag; // Variable to check if a palindrome is found
for (var i = new_str.length;; i++) { // Loop to increment the length for building a palindrome
flag = true; // Set the flag initially to true for each iteration
for (var j = 0; j < i - j - 1; j++) { // Loop to check for palindrome condition
// Check if characters symmetrically positioned around the center are equal
if (i - j - 1 < new_str.length && new_str[j] != new_str[i - j - 1]) {
flag = false; // Set flag to false if characters are not equal
break; // Break the loop if not a palindrome
}
}
if (flag) {
// If a palindrome is found, complete the palindrome by adding the remaining characters
for (var j = new_str.length; j < i; j++) {
new_str += new_str[i - j - 1];
}
return new_str; // Return the palindrome
}
}
}
console.log(build_Palindrome("abcddc")); // Output the result for the given string
console.log(build_Palindrome("122")); // Output the result for the given string
Output:
abcddcba 1221
Live Demo:
See the Pen javascript-basic-exercise-97 by w3resource (@w3resource) on CodePen.
Flowchart:
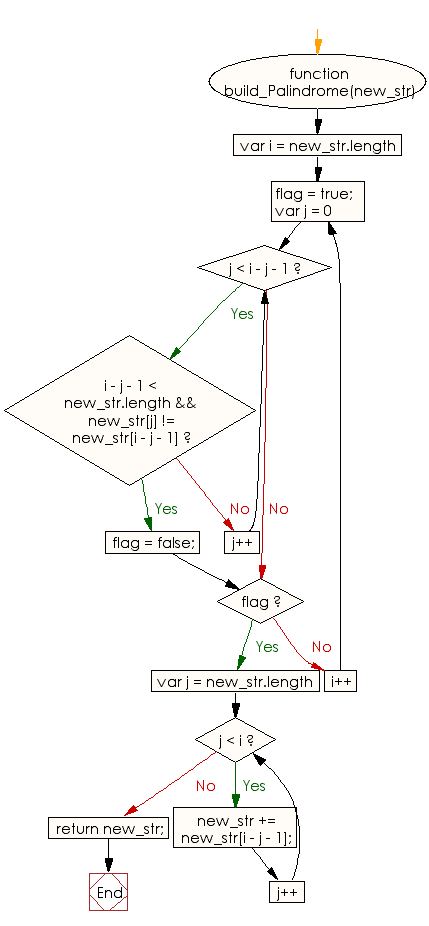
ES6 Version:
// Function to build a palindrome from a given string
const build_Palindrome = (new_str) => {
let flag; // Variable to check if a palindrome is found
for (let i = new_str.length;; i++) { // Infinite loop starting from the length of the input string
flag = true; // Reset the flag for each iteration
for (let j = 0; j < i - j - 1; j++) {
// Check if the characters symmetrically positioned around the center are equal
if (i - j - 1 < new_str.length && new_str[j] !== new_str[i - j - 1]) {
flag = false; // Set the flag to false if not equal
break; // Break the inner loop
}
}
if (flag) {
// If a palindrome is found, complete the palindrome by adding the remaining characters
for (let j = new_str.length; j < i; j++) {
new_str += new_str[i - j - 1];
}
return new_str; // Return the palindrome
}
}
}
console.log(build_Palindrome("abcddc")); // Example usage
console.log(build_Palindrome("122")); // Example usage
For more Practice: Solve these Related Problems:
- Write a JavaScript program that finds the shortest string which, when appended to the given string, transforms it into a palindrome.
- Write a JavaScript function that calculates the minimal suffix needed to convert a given string into a palindrome.
- Write a JavaScript program that determines and returns the shortest possible string that can be concatenated to the original string to form a palindrome.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to compute the sum of absolute differences of consecutive numbers of a given array of integers.
Next: JavaScript program to switch case of the minimum possible number of letters to make a given string written in the upper case or in the lower case.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics