JavaScript: Compute the sum of absolute differences of consecutive numbers of a given array of integers
JavaScript Basic: Exercise-96 with Solution
Sum of Absolute Differences of Consecutive Numbers
Write a JavaScript program to compute the sum of the absolute differences of consecutive numbers in a given array of integers.
Visual Presentation:
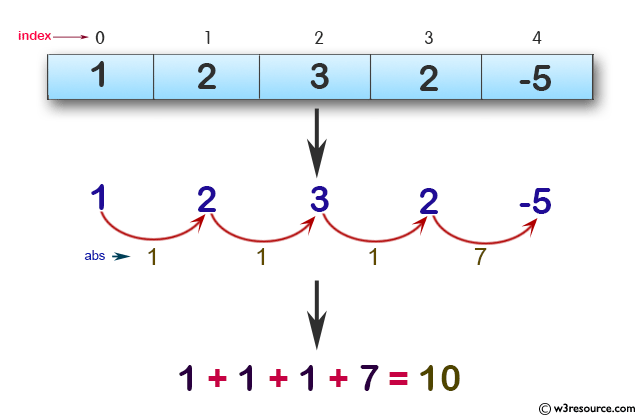
Sample Solution:
JavaScript Code:
// Function to calculate the sum of adjacent differences in an array
function sum_adjacent_difference(arr) {
var result = 0; // Variable to store the result
for (var i = 1; i < arr.length; i++) {
// Add the absolute difference between adjacent elements to the result
result += Math.abs(arr[i] - arr[i - 1]);
}
return result; // Return the final sum
}
console.log(sum_adjacent_difference([1, 2, 3, 2, -5])); // Example usage
Output:
10
Live Demo:
See the Pen javascript-basic-exercise-96 by w3resource (@w3resource) on CodePen.
Flowchart:
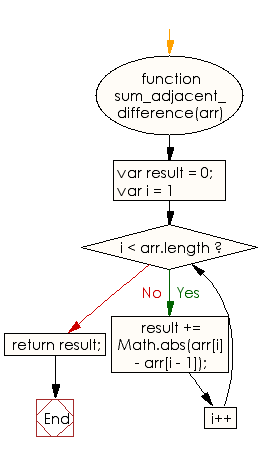
ES6 Version:
// Function to calculate the sum of absolute differences between adjacent elements in an array
const sum_adjacent_difference = (arr) => {
let result = 0; // Initialize the result variable to store the sum
// Iterate through the array starting from the second element
for (let i = 1; i < arr.length; i++) {
result += Math.abs(arr[i] - arr[i - 1]); // Add the absolute difference to the result
}
return result; // Return the final sum
};
console.log(sum_adjacent_difference([1, 2, 3, 2, -5])); // Example usage
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to replace all the numbers with a specified number of a given array of integers.
Next: JavaScript program to find the shortest possible string which can create a string to make it a palindrome by adding characters to the end of it.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics