JavaScript: Replace all the numbers with a specified number of a given array of integers
JavaScript Basic: Exercise-95 with Solution
Replace Numbers with Specified Value in Array
Write a JavaScript program to replace all numbers with a specified number in an array of integers.
Visual Presentation:
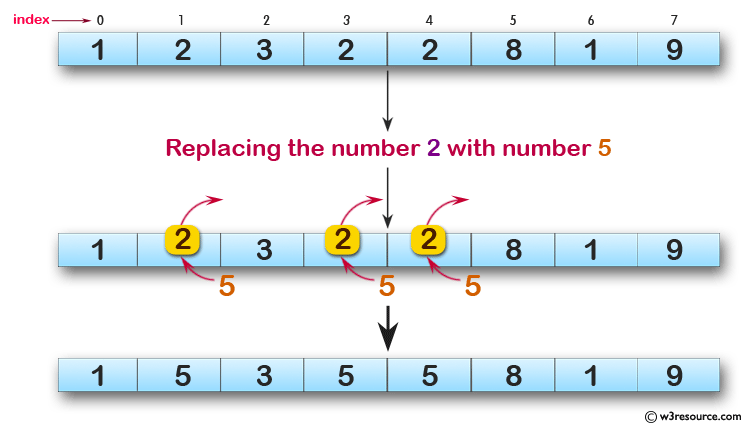
Sample Solution:
JavaScript Code:
// Function to replace a specified element in an array with a new value
function array_element_replace(arr, old_value, new_value) {
for (var i = 0; i < arr.length; i++) {
// Check if the current element is equal to the old value
if (arr[i] === old_value) {
arr[i] = new_value; // Replace the old value with the new value
}
}
return arr; // Return the modified array
}
// Example usage
num = [1, 2, 3, 2, 2, 8, 1, 9]; // Original array
console.log("Original Array: " + num);
console.log(array_element_replace(num, 2, 5)); // Replace 2 with 5 in the array
Output:
Original Array: 1,2,3,2,2,8,1,9 [1,5,3,5,5,8,1,9]
Live Demo:
See the Pen javascript-basic-exercise-95 by w3resource (@w3resource) on CodePen.
Flowchart:
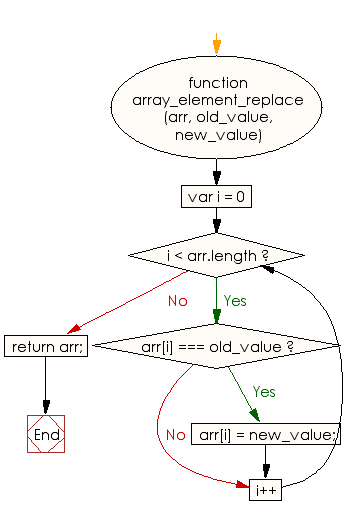
ES6 Version:
// Function to replace all occurrences of a specified value in an array
const array_element_replace = (arr, old_value, new_value) => {
// Iterate through the array
for (let i = 0; i < arr.length; i++) {
// Check if the current element is equal to the old value
if (arr[i] === old_value) {
arr[i] = new_value; // Replace with the new value
}
}
return arr; // Return the modified array
};
let num = [1, 2, 3, 2, 2, 8, 1, 9]; // Example array
console.log("Original Array: " + num);
console.log(array_element_replace(num, 2, 5));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that replaces every element in an array with a specified value provided as input.
- Write a JavaScript function that maps an array of numbers to a new array where each element is replaced by a constant value.
- Write a JavaScript program that creates a copy of an array and then substitutes all its elements with a given number, preserving the original array.
Go to:
PREV : Find Most Frequent Number in Array.
NEXT : Sum of Absolute Differences of Consecutive Numbers.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.