JavaScript: Find the number which appears most in a given array of integers
JavaScript Basic: Exercise-94 with Solution
Find Most Frequent Number in Array
Write a JavaScript program to find the number appearing most frequently in a given array of integers.
Visual Presentation:
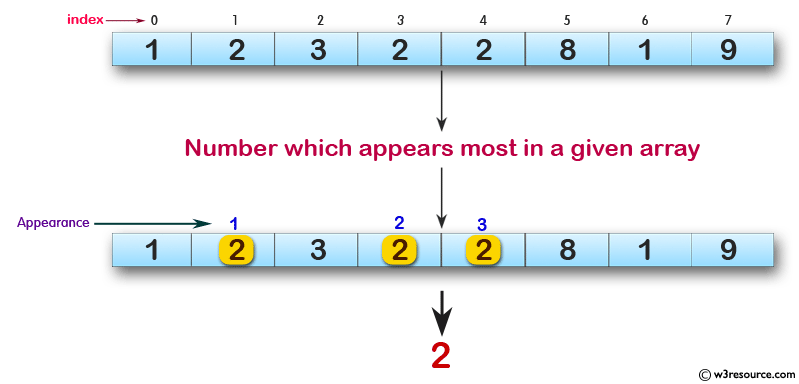
Sample Solution:
JavaScript Code:
// Function to find the mode (most frequently occurring element) in an array
function array_element_mode(arr) {
var ctr = [], // Counter array to store frequencies of elements
ans = 0; // Variable to store the index of the mode
// Initialize the counter array with zeros for each possible element
for (var i = 0; i < 10; i++) {
ctr.push(0);
}
// Iterate through the input array to update the frequencies in the counter array
for (var i = 0; i < arr.length; i++) {
ctr[arr[i] - 1]++; // Increment the frequency of the current element
if (ctr[arr[i] - 1] > ctr[ans]) {
ans = arr[i] - 1; // Update the index of the mode if a higher frequency is found
}
}
return ans + 1; // Return the mode (add 1 to convert from zero-based index to element value)
}
// Example usage
console.log(array_element_mode([1, 2, 3, 2, 2, 8, 1, 9])); // 2
Output:
2
Live Demo:
See the Pen javascript-basic-exercise-94 by w3resource (@w3resource) on CodePen.
Flowchart:
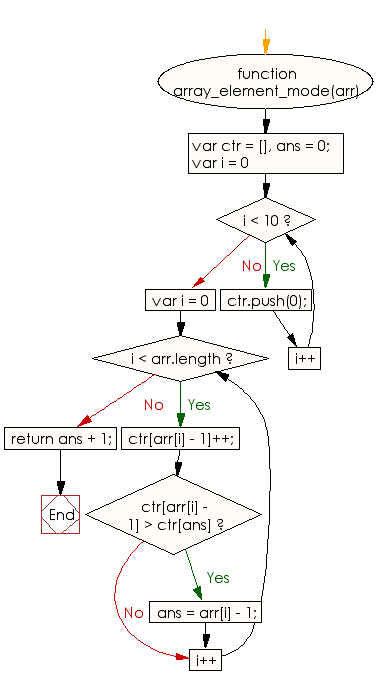
ES6 Version:
// Function to find the mode (most frequent element) in an array
const array_element_mode = (arr) => {
let ctr = []; // Array to store the count of each element
let ans = 0; // Variable to store the index with the highest count
// Initialize the count array with zeros for each possible element (1 to 10)
for (let i = 0; i < 10; i++) {
ctr.push(0);
}
// Iterate through the input array to count occurrences of each element
for (let i = 0; i < arr.length; i++) {
ctr[arr[i] - 1]++; // Increment the count for the corresponding element
if (ctr[arr[i] - 1] > ctr[ans]) {
ans = arr[i] - 1; // Update the index with the highest count
}
}
return ans + 1; // Return the mode (element with the highest count)
};
// Example usage
console.log(array_element_mode([1, 2, 3, 2, 2, 8, 1, 9]));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that builds a frequency map from an array of integers and returns the number that appears most frequently.
- Write a JavaScript function that identifies the mode in an array of numbers, handling cases with multiple modes by returning the smallest.
- Write a JavaScript program that iterates through an array to determine the most common number, and returns both the number and its frequency.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to find the maximum difference among all possible pairs of a given array of integers.
Next: JavaScript program to replace all the numbers with a specified number of a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.